def create_frustum(self): # Create grid in image plane h, w = self.cfg.IMAGE.FINAL_DIM downsampled_h, downsampled_w = h // self.encoder_downsample, w // self.encoder_downsample # Depth grid depth_grid = torch.arange(*self.cfg.LIFT.D_BOUND, dtype=torch.float) depth_grid = depth_grid.view(-1, 1, 1).expand(-1, downsampled_h, downsampled_w) n_depth_slices = depth_grid.shape[0] # x and y grids x_grid = torch.linspace(0, w - 1, downsampled_w, dtype=torch.float) x_grid = x_grid.view(1, 1, downsampled_w).expand(n_depth_slices, downsampled_h, downsampled_w) y_grid = torch.linspace(0, h - 1, downsampled_h, dtype=torch.float) y_grid = y_grid.view(1, downsampled_h, 1).expand(n_depth_slices, downsampled_h, downsampled_w) # Dimension (n_depth_slices, downsampled_h, downsampled_w, 3) # containing data points in the image: left-right, top-bottom, depth frustum = torch.stack((x_grid, y_grid, depth_grid), -1) return nn.Parameter(frustum, requires_grad=False)这里的frustum可以可视化吗?
时间: 2024-03-23 09:35:51 浏览: 109
可以的,你可以使用Matplotlib库将`frustum`可视化。以下是一个简单的示例代码,可以将`frustum`中的数据点可视化为散点图:
```python
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 将frustum转换为Numpy数组
frustum_np = frustum.detach().cpu().numpy()
# 创建散点图
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(frustum_np[:, :, :, 0].flatten(),
frustum_np[:, :, :, 1].flatten(),
frustum_np[:, :, :, 2].flatten(),
s=1)
# 设置坐标轴标签
ax.set_xlabel('x')
ax.set_ylabel('y')
ax.set_zlabel('depth')
# 显示图像
plt.show()
```
这将显示一个三维散点图,其中x轴和y轴分别对应于图像中的水平和垂直坐标,z轴对应于深度值。
相关问题
def create_frustum(self): # Create grid in image plane h, w = self.cfg.IMAGE.FINAL_DIM downsampled_h, downsampled_w = h // self.encoder_downsample, w // self.encoder_downsample # Depth grid depth_grid = torch.arange(*self.cfg.LIFT.D_BOUND, dtype=torch.float) depth_grid = depth_grid.view(-1, 1, 1).expand(-1, downsampled_h, downsampled_w) n_depth_slices = depth_grid.shape[0] # x and y grids x_grid = torch.linspace(0, w - 1, downsampled_w, dtype=torch.float) x_grid = x_grid.view(1, 1, downsampled_w).expand(n_depth_slices, downsampled_h, downsampled_w) y_grid = torch.linspace(0, h - 1, downsampled_h, dtype=torch.float) y_grid = y_grid.view(1, downsampled_h, 1).expand(n_depth_slices, downsampled_h, downsampled_w) # Dimension (n_depth_slices, downsampled_h, downsampled_w, 3) # containing data points in the image: left-right, top-bottom, depth frustum = torch.stack((x_grid, y_grid, depth_grid), -1) return nn.Parameter(frustum, requires_grad=False)最终得到的frustum是几维的
最终得到的frustum是4维的,维度为 (n_depth_slices, downsampled_h, downsampled_w, 3)。其中n_depth_slices是深度网格的数量,downsampled_h和downsampled_w是图像经过下采样后的高度和宽度,3表示每个数据点的坐标分别为x、y和深度。
def get_geometry(self, intrinsics, extrinsics): """Calculate the (x, y, z) 3D position of the features. """ rotation, translation = extrinsics[..., :3, :3], extrinsics[..., :3, 3] B, N, _ = translation.shape # Add batch, camera dimension, and a dummy dimension at the end points = self.frustum.unsqueeze(0).unsqueeze(0).unsqueeze(-1) # Camera to ego reference frame points = torch.cat((points[:, :, :, :, :, :2] * points[:, :, :, :, :, 2:3], points[:, :, :, :, :, 2:3]), 5) combined_transformation = rotation.matmul(torch.inverse(intrinsics)) points = combined_transformation.view(B, N, 1, 1, 1, 3, 3).matmul(points).squeeze(-1) points += translation.view(B, N, 1, 1, 1, 3) # The 3 dimensions in the ego reference frame are: (forward, sides, height) return points这段代码的意思是什么?
这段代码是一个用于将二维图像特征点映射到三维空间中的函数。它接收相机内参和外参作为输入,并返回每个特征点的三维坐标。
在函数中,首先从外参中提取旋转和平移矩阵。然后将输入的二维特征点扩展成一个四维张量,其中第一维是batch size,第二维是相机数量,第三维是特征点数量,第四维是x、y和一个占位的维度。
接下来,通过一系列的数学运算将特征点从相机坐标系转换到自车坐标系。这里的转换包括以下几个步骤:
1. 将二维特征点的前两个维度(x和y)乘以深度(z)并将其放在新的第三个维度中,这样就得到了三维特征点的相机坐标。
2. 将相机坐标转换为自车参考系下的坐标。这里使用了相机内参矩阵和旋转矩阵进行计算。
3. 将平移矩阵加到每个特征点的位置上,以获得相对于自车坐标系的绝对位置。
最后,返回每个特征点的三维坐标。
阅读全文
相关推荐










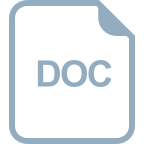

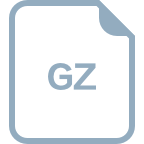



