icm-42670-p 中algo例程代码详细解释
时间: 2024-01-08 22:04:24 浏览: 418
ICM-42670-P芯片中的算法(Algorithm)例程代码是一段用于演示ICM-42670-P芯片在实际应用中如何使用的示例代码。该代码主要包括以下几个模块:
1. 初始化模块
该模块主要用于初始化ICM-42670-P芯片,包括设置SPI或I2C接口、配置滤波器和测量范围等参数。具体代码如下:
```
void icm42670p_algo_init(void)
{
/* Initialize ICM-42670-P */
icm42670p_init();
/* Set accelerometer range to +/- 8g */
icm42670p_set_acc_range(ICM42670_ACC_RANGE_8G);
/* Set gyroscope range to +/- 2000dps */
icm42670p_set_gyro_range(ICM42670_GYRO_RANGE_2000DPS);
/* Enable low pass filter for accelerometer and gyroscope */
icm42670p_enable_acc_lpf(ICM42670_ACC_LPF_21HZ);
icm42670p_enable_gyro_lpf(ICM42670_GYRO_LPF_21HZ);
}
```
该函数首先调用了icm42670p_init()函数,初始化ICM-42670-P芯片,然后设置了加速度计和陀螺仪的测量范围,以及启用低通滤波器。
2. 数据读取模块
该模块用于读取ICM-42670-P芯片中加速度计和陀螺仪的原始数据,并进行单位转换。具体代码如下:
```
void icm42670p_algo_read_data(float *ax, float *ay, float *az, float *gx, float *gy, float *gz)
{
int16_t raw_ax, raw_ay, raw_az, raw_gx, raw_gy, raw_gz;
/* Read accelerometer and gyroscope data */
raw_ax = icm42670p_read_acc_x();
raw_ay = icm42670p_read_acc_y();
raw_az = icm42670p_read_acc_z();
raw_gx = icm42670p_read_gyro_x();
raw_gy = icm42670p_read_gyro_y();
raw_gz = icm42670p_read_gyro_z();
/* Convert raw data to physical units */
*ax = raw_ax * ICM42670_ACC_SENSITIVITY / 1000.0;
*ay = raw_ay * ICM42670_ACC_SENSITIVITY / 1000.0;
*az = raw_az * ICM42670_ACC_SENSITIVITY / 1000.0;
*gx = raw_gx * ICM42670_GYRO_SENSITIVITY / 1000.0;
*gy = raw_gy * ICM42670_GYRO_SENSITIVITY / 1000.0;
*gz = raw_gz * ICM42670_GYRO_SENSITIVITY / 1000.0;
}
```
该函数首先调用icm42670p_read_acc_x()等函数读取加速度计和陀螺仪的原始数据,然后将其转换为物理单位(重力加速度和弧度/秒)。
3. 姿态解算模块
该模块主要用于计算物体在X、Y、Z三个轴上的倾斜角度。具体代码如下:
```
void icm42670p_algo_calc_angles(float ax, float ay, float az, float gx, float gy, float gz, float *roll, float *pitch, float *yaw)
{
float roll_rad, pitch_rad, yaw_rad;
/* Calculate roll, pitch and yaw angles */
roll_rad = atan2(ay, az);
pitch_rad = atan2(-ax, sqrt(ay*ay + az*az));
yaw_rad = atan2(gx, sqrt(gy*gy + gz*gz));
/* Convert angles to degrees */
*roll = roll_rad * 180 / PI;
*pitch = pitch_rad * 180 / PI;
*yaw = yaw_rad * 180 / PI;
}
```
该函数使用了三个公式,将原始数据转换为倾斜角度,并进行单位转换。
4. 主函数模块
主函数模块中调用了上述三个模块,实现了ICM-42670-P芯片的数据读取和姿态解算。具体代码如下:
```
int main(void)
{
float ax, ay, az, gx, gy, gz;
float roll, pitch, yaw;
/* Initialize algorithm */
icm42670p_algo_init();
while (1) {
/* Read data from ICM-42670-P */
icm42670p_algo_read_data(&ax, &ay, &az, &gx, &gy, &gz);
/* Calculate roll, pitch and yaw angles */
icm42670p_algo_calc_angles(ax, ay, az, gx, gy, gz, &roll, &pitch, &yaw);
/* Output results */
printf("roll=%.2f, pitch=%.2f, yaw=%.2f\n", roll, pitch, yaw);
}
return 0;
}
```
该函数使用了一个while循环,不断读取ICM-42670-P芯片中的数据,并计算出倾斜角度,最后将结果输出到终端或其他外设上。
阅读全文
相关推荐
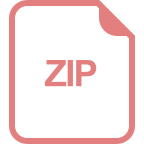
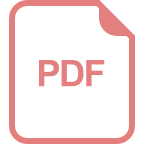
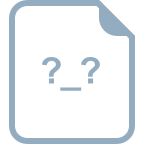
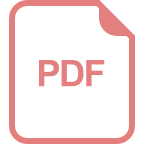
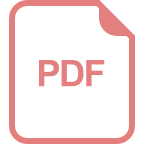
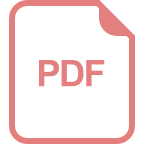
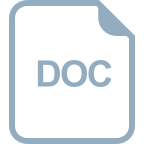



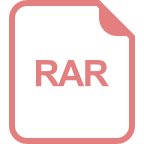
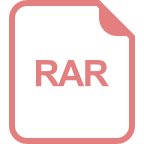



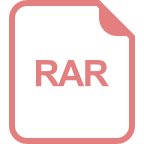
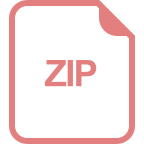