写出c语言代码(用指针实现):在主函数中输入10个等长的字符串。用另一函数对它们排序。然后在主函数输出这10个已排好序的字符串。
时间: 2024-03-09 19:44:52 浏览: 19
好的,这是一个用指针实现字符串排序的C语言代码,希望对您有所帮助:
```c
#include <stdio.h>
#include <string.h>
void sort(char *str[], int n);
int main()
{
char *str[10];
int i;
printf("请输入10个等长的字符串:\n");
for (i = 0; i < 10; i++) {
char temp[100];
scanf("%s", temp);
str[i] = (char*)malloc(strlen(temp) + 1);
strcpy(str[i], temp);
}
sort(str, 10);
printf("排序后的字符串为:\n");
for (i = 0; i < 10; i++) {
printf("%s\n", str[i]);
}
return 0;
}
void sort(char *str[], int n)
{
int i, j;
char *temp;
for (i = 0; i < n - 1; i++) {
for (j = i + 1; j < n; j++) {
if (strcmp(str[i], str[j]) > 0) {
temp = str[i];
str[i] = str[j];
str[j] = temp;
}
}
}
}
```
解释一下,我们首先在主函数中定义一个指向字符指针的数组 `char *str[10]` 来存储10个字符串,然后通过 `scanf` 函数输入字符串并使用 `malloc` 函数在内存中为每个字符串分配空间。接下来,我们调用 `sort` 函数来对这些字符串进行排序。排序完成后,我们再在主函数中输出排好序的字符串。
在 `sort` 函数中,我们使用冒泡排序算法来对字符串进行比较和交换。由于 `str` 数组是一个指向字符指针的数组,我们在比较和交换字符串时只需要操作指针即可。
希望这段代码能帮助您理解如何用指针实现字符串排序。
相关推荐
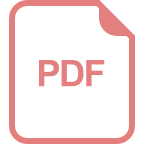
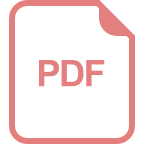













