编程:已有抽象类shape定义如下,该类提供三个纯虚函数;显示数据成员、返回面积和返回体积。 Class Shape { virtual void showData()=0; virtual double reArea()=0; virtual double reVolume()=0; }; 以Shape为顶层基类,派生TwoDimShape(二维图形)和ThreeShape(三维图形)两个类,它们增加了有关的数据成员(自己设定,各自不少于2个),但没有成员函数的实现。 第三层派生具体的图形类。TwoDimShape类派生Circle(圆)、Elipse(椭圆)、Rectangle(矩形)和Triangle(三角形)等类。ThreeShape类派生Ball(球体)、Cylinder(圆柱体)、RectangularParallelepiped(长方体)等类。 在主函数测试中使用多态方式调用不同对象的求面积和体积等函数。
时间: 2024-01-17 18:06:10 浏览: 70
好的,下面是一个可能的实现,供参考:
```c++
#include <iostream>
#include <cmath>
using namespace std;
// 抽象类 Shape
class Shape {
public:
virtual void showData() = 0; // 显示数据成员
virtual double reArea() = 0; // 返回面积
virtual double reVolume() = 0; // 返回体积
};
// 二维图形类 TwoDimShape
class TwoDimShape : public Shape {
public:
TwoDimShape() {}
virtual ~TwoDimShape() {}
protected:
double width; // 宽度
double height; // 高度
};
// 三维图形类 ThreeShape
class ThreeShape : public Shape {
public:
ThreeShape() {}
virtual ~ThreeShape() {}
protected:
double width; // 宽度
double height; // 高度
double depth; // 深度
};
// 圆形类 Circle
class Circle : public TwoDimShape {
public:
Circle(double r) : radius(r) {}
virtual ~Circle() {}
virtual void showData() {
cout << "Circle: radius = " << radius << endl;
}
virtual double reArea() {
return M_PI * radius * radius;
}
virtual double reVolume() {
return 0.0;
}
private:
double radius; // 半径
};
// 椭圆类 Elipse
class Elipse : public TwoDimShape {
public:
Elipse(double a, double b) : a_axis(a), b_axis(b) {}
virtual ~Elipse() {}
virtual void showData() {
cout << "Elipse: a-axis = " << a_axis << ", b-axis = " << b_axis << endl;
}
virtual double reArea() {
return M_PI * a_axis * b_axis;
}
virtual double reVolume() {
return 0.0;
}
private:
double a_axis; // 长半轴
double b_axis; // 短半轴
};
// 矩形类 Rectangle
class Rectangle : public TwoDimShape {
public:
Rectangle(double w, double h) : width(w), height(h) {}
virtual ~Rectangle() {}
virtual void showData() {
cout << "Rectangle: width = " << width << ", height = " << height << endl;
}
virtual double reArea() {
return width * height;
}
virtual double reVolume() {
return 0.0;
}
};
// 三角形类 Triangle
class Triangle : public TwoDimShape {
public:
Triangle(double w, double h) : width(w), height(h) {}
virtual ~Triangle() {}
virtual void showData() {
cout << "Triangle: width = " << width << ", height = " << height << endl;
}
virtual double reArea() {
return width * height / 2.0;
}
virtual double reVolume() {
return 0.0;
}
};
// 球体类 Ball
class Ball : public ThreeShape {
public:
Ball(double r) : radius(r) {}
virtual ~Ball() {}
virtual void showData() {
cout << "Ball: radius = " << radius << endl;
}
virtual double reArea() {
return 4.0 * M_PI * radius * radius;
}
virtual double reVolume() {
return 4.0 / 3.0 * M_PI * radius * radius * radius;
}
private:
double radius; // 半径
};
// 圆柱体类 Cylinder
class Cylinder : public ThreeShape {
public:
Cylinder(double r, double h) : radius(r), height(h) {}
virtual ~Cylinder() {}
virtual void showData() {
cout << "Cylinder: radius = " << radius << ", height = " << height << endl;
}
virtual double reArea() {
return 2.0 * M_PI * radius * height + 2.0 * M_PI * radius * radius;
}
virtual double reVolume() {
return M_PI * radius * radius * height;
}
private:
double radius; // 半径
double height; // 高度
};
// 长方体类 RectangularParallelepiped
class RectangularParallelepiped : public ThreeShape {
public:
RectangularParallelepiped(double w, double h, double d) : width(w), height(h), depth(d) {}
virtual ~RectangularParallelepiped() {}
virtual void showData() {
cout << "RectangularParallelepiped: width = " << width << ", height = " << height << ", depth = " << depth << endl;
}
virtual double reArea() {
return 2.0 * (width * height + height * depth + depth * width);
}
virtual double reVolume() {
return width * height * depth;
}
private:
double width; // 宽度
double height; // 高度
double depth; // 深度
};
int main() {
Shape* shapes[] = {
new Circle(2.0),
new Elipse(2.0, 3.0),
new Rectangle(2.0, 3.0),
new Triangle(2.0, 3.0),
new Ball(2.0),
new Cylinder(2.0, 3.0),
new RectangularParallelepiped(2.0, 3.0, 4.0)
};
int n = sizeof(shapes) / sizeof(shapes[0]);
for (int i = 0; i < n; i++) {
shapes[i]->showData();
cout << "Area = " << shapes[i]->reArea() << endl;
cout << "Volume = " << shapes[i]->reVolume() << endl;
cout << endl;
}
for (int i = 0; i < n; i++) {
delete shapes[i];
}
return 0;
}
```
在这个实现中,我们先定义了抽象类`Shape`,其中包含三个纯虚函数。然后定义了`TwoDimShape`和`ThreeShape`两个派生类,用于表示二维图形和三维图形。这两个类都增加了自己的数据成员。接着,我们定义了一些具体的图形类,如`Circle`、`Elipse`、`Rectangle`、`Triangle`、`Ball`、`Cylinder`和`RectangularParallelepiped`等,它们分别继承自`TwoDimShape`和`ThreeShape`类,并实现了自己的成员函数。
在主函数中,我们定义了一个`Shape`类型的数组,用于存放不同的图形对象。然后,使用多态的方式,依次调用每个对象的成员函数,计算面积和体积,并输出结果。最后,释放对象的内存。
阅读全文
相关推荐
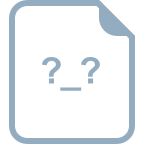
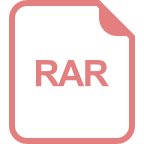














