Code provides features that may automatically execute files in this folder. If you don't trust the authors of these files, we recommend to continue in restricted mode as the files may be malicious. See our docs to learn more.
时间: 2024-03-11 11:45:01 浏览: 141
这是一条代码扫描工具的警告信息,意思是说这个文件夹中的文件可能会自动执行某些操作,如果你不信任文件的作者,建议以受限制的模式继续操作,因为这些文件可能是恶意的。你可以查看相关文档以了解更多信息。
一般来说,如果你知道这些文件的来源并信任其作者,可以选择信任并继续操作,否则建议以受限制的模式继续操作,以避免安全风险。
相关问题
Create a file writing class Create a new class that writes to the included txt file. Use a BufferedWriter to write the following text to the file: What took you so long? Well, you know, Master, I couldn't find a speeder that I really liked... There he is. ...with an open cockpit and the right speed capabilities. If you spent as much time practicing your saber techniques as you did your wit, you'd rival Master Yoda as a swordsman. I thought I already did. Only in your mind, my very young apprentice. Make sure that all the line breaks are correct. Hint: You can access the file via its relative path by just stating its name: conversation.txt. No need to have the complete path to the file.
Sure, here's an example code for the requested class:
```java
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
public class FileWriterClass {
public static void writeToFile() {
try {
BufferedWriter writer = new BufferedWriter(new FileWriter("conversation.txt"));
writer.write("What took you so long?\n");
writer.write("Well, you know, Master, I couldn't find a speeder that I really liked...\n");
writer.write("There he is.\n");
writer.write("...with an open cockpit and the right speed capabilities.\n");
writer.write("If you spent as much time practicing your saber techniques as you did your wit, you'd rival Master Yoda as a swordsman.\n");
writer.write("I thought I already did.\n");
writer.write("Only in your mind, my very young apprentice.\n");
writer.close();
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
```
This class includes a `writeToFile()` method that creates a `BufferedWriter` object and writes the given text to the specified file name `conversation.txt`. The `BufferedWriter` handles the line breaks automatically, and the code catches and prints any possible IO errors that may occur during the writing process.
You can call this method from anywhere in your code by simply invoking `FileWriterClass.writeToFile()`.
1.Discuss how and why the SecurityManager class for JVM check methods can be defined differently based on context.. 2.The library class Collections contains a method sort with the following type signature. public static <T> void sort (T[] a, Comparator <? super T> c); The Comparator interface contains the following method which returns a negative integer, zero or a positive integer according to whether o1 is considered to be before, equal to or after o2 in a proposed ordering. int compare (T o1, T o2) ; What does the identifier T mean in the declaration of the method sort? Illustrate your answer with an example. 3.What does the notation <? super T> mean in the declaration of sort? Again, illustrate your answer with an example. 4.Explain the concept of an anonymous inner class in Java using a method call example. 5.Convert the method call example using an anonymous inner class to a lambda expression. Give the code required for this. 6.In what types of situations might you prefer to use an anonymous class instead of a lambda expression, and vice versa? 7.Explain (auto)boxing and (auto)unboxing in Java? 8.What does the annotation @Override mean in Java? What are the benefits of adding it? 9.What are wildcards in Java and how are they used in generic programming? 10.Can you explain the bounded wildcards, and provide an example
1. The SecurityManager class for JVM check methods can be defined differently based on context because different contexts may have different security requirements and restrictions. For example, a web application may need to restrict access to certain resources, while a desktop application may not have the same restrictions.
2. The identifier T in the declaration of the method sort represents a generic type parameter that can be replaced with any type when the method is called. For example, if we have an array of integers, we can call the sort method like this:
```
Integer[] arr = {3, 1, 4, 1, 5, 9, 2, 6, 5, 3};
Comparator<Integer> c = Comparator.naturalOrder();
Collections.sort(arr, c);
```
3. The notation <? super T> in the declaration of sort means that the Comparator can accept any type that is a superclass of T. For example, if we have a class Animal and a subclass Dog, we can use a Comparator that accepts any superclass of Dog to sort an array of Dogs:
```
Dog[] dogs = ...;
Comparator<Animal> c = ...;
Collections.sort(dogs, c);
```
4. An anonymous inner class in Java is a class that is defined and instantiated at the same time, without a separate class definition. For example, we can define an anonymous inner class to implement an interface and pass it as an argument to a method:
```
Runnable r = new Runnable() {
public void run() {
System.out.println("Hello, world!");
}
};
r.run();
```
5. The same example using a lambda expression would look like this:
```
Runnable r = () -> System.out.println("Hello, world!");
r.run();
```
6. You might prefer to use an anonymous class instead of a lambda expression when you need to define a class that has additional behavior or state beyond the method being called. You might prefer to use a lambda expression when you only need to define a single method and want to keep the code more concise.
7. (Auto)boxing and (auto)unboxing in Java refer to the automatic conversion between primitive types and their corresponding object wrapper types. For example, an int can be automatically boxed to an Integer, and an Integer can be automatically unboxed to an int.
8. The annotation @Override in Java indicates that a method is intended to override a method in a superclass or interface. Adding this annotation can help catch errors at compile time and make the code more readable.
9. Wildcards in Java are used in generic programming to allow a generic type to accept any type that meets a certain criteria. For example, a List<? extends Number> can accept a List of any subclass of Number.
10. Bounded wildcards in Java restrict the types that can be used with a generic type parameter. For example, a List<? extends Number> can only accept types that are subclasses of Number, while a List<? super Integer> can accept types that are superclasses of Integer. An example of using a bounded wildcard would be:
```
public static double sum(List<? extends Number> list) {
double total = 0.0;
for (Number n : list) {
total += n.doubleValue();
}
return total;
}
```
阅读全文
相关推荐
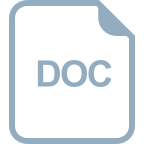
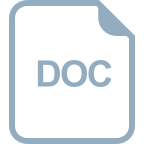
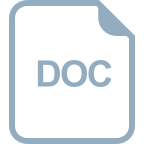
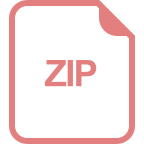
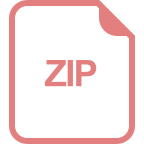
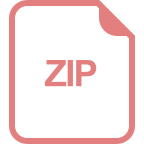
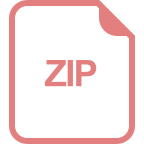
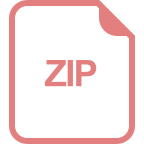
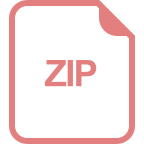
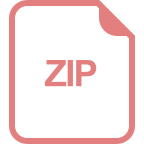
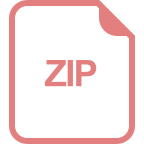
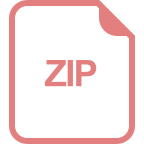
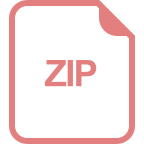
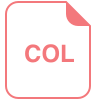



