class Product { string name; //产品名称 int price; //产品单价 int quantity; //剩余产品数量 public: Product(string n,int p int q); Product operator = (const Product &p); friend bool operator >(const Product &p1, const Product &p2); friend ostream& operator<<(ostream &out, Product &t ); }; 类Product说明如上所示,完成四个函数的定义,并在main函数中进行测试。
时间: 2024-02-14 11:12:57 浏览: 57
下面是类 `Product` 的四个函数的定义:
```c++
#include <iostream>
using namespace std;
class Product {
string name; // 产品名称
int price; // 产品单价
int quantity; // 剩余产品数量
public:
Product(string n, int p, int q) : name(n), price(p), quantity(q) {} // 构造函数
// 赋值运算符重载
Product operator=(const Product &p) {
name = p.name;
price = p.price;
quantity = p.quantity;
return *this;
}
// 大于运算符重载
friend bool operator>(const Product &p1, const Product &p2) {
return p1.price > p2.price;
}
// 流插入运算符重载
friend ostream& operator<<(ostream &out, Product &t) {
out << "名称:" << t.name << ",单价:" << t.price << ",数量:" << t.quantity;
return out;
}
};
int main() {
Product p1("产品1", 20, 10);
Product p2("产品2", 30, 5);
if (p1 > p2) {
cout << "p1的单价更高" << endl;
} else {
cout << "p2的单价更高" << endl;
}
cout << "产品1:" << p1 << endl;
cout << "产品2:" << p2 << endl;
Product p3 = p1;
cout << "产品3(通过赋值得到):" << p3 << endl;
return 0;
}
```
上述代码中,首先定义了 `Product` 类,其中包含了三个成员变量 `name`、`price` 和 `quantity`,分别代表产品的名称、单价和剩余数量。接着,定义了类的构造函数,用于在创建对象时初始化成员变量。
然后,重载了赋值运算符 `operator=`,用于实现对象之间的赋值操作。由于该函数需要修改当前对象的成员变量,因此使用了 `this` 指针。
接着,重载了大于运算符 `operator>`,用于比较两个 `Product` 对象的单价大小。
最后,重载了流插入运算符 `operator<<`,用于将 `Product` 对象输出到流中,以便于显示。
在 `main()` 函数中,创建了两个 `Product` 对象 `p1` 和 `p2`,并比较了它们的单价大小。然后,通过调用赋值运算符重载函数将 `p1` 对象的值赋给了 `p3` 对象,并将三个对象的信息输出到标准输出流中。
阅读全文
相关推荐
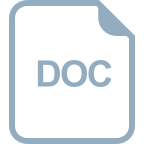
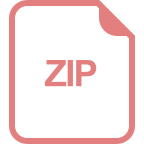







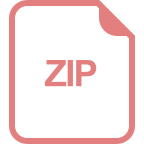
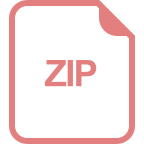
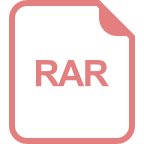
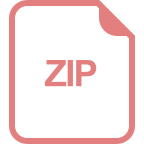
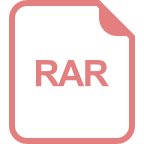
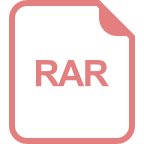
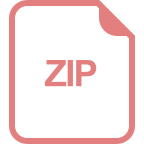
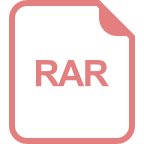