爬取链家成都市2022和2023的租房房源数据,包括房源名称", "户型", "面向", "面积", "所属区", "地址", "价钱"
时间: 2023-10-16 11:10:35 浏览: 47
好的,这个任务需要使用爬虫技术,你需要先安装 Python 环境和相关的库,比如 requests、beautifulsoup4 和 pandas。然后,你可以使用 requests 库发送 HTTP 请求,获取链家网站的 HTML 页面,再使用 beautifulsoup4 库解析 HTML 页面,提取出需要的数据。最后,你可以使用 pandas 库将数据保存到 Excel 文件中。
以下是示例代码:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 设置请求头
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 定义函数,用于获取指定页面的房源数据
def get_house_data(url):
# 发送 HTTP 请求
response = requests.get(url, headers=headers)
# 解析 HTML 页面
soup = BeautifulSoup(response.text, 'html.parser')
# 获取房源列表
house_list = soup.find_all('div', class_='content__list--item--main')
# 遍历房源列表,提取数据
data = []
for house in house_list:
name = house.find('p', class_='content__list--item--title').text.strip()
layout = house.find('p', class_='content__list--item--des').text.strip()
orientation = house.find_all('p', class_='content__list--item--des')[1].text.strip()
area = house.find_all('p', class_='content__list--item--des')[2].text.strip()
district = house.find('p', class_='content__list--item--neighborhood').text.strip()
address = house.find('p', class_='content__list--item--address').text.strip()
price = house.find('span', class_='content__list--item-price').text.strip()
data.append([name, layout, orientation, area, district, address, price])
return data
# 定义函数,用于获取指定区域和时间段的所有房源数据
def get_all_house_data(district, start_year, end_year):
# 定义 URL 模板
url_template = 'https://cd.lianjia.com/zufang/{}/pg{}{}'
# 定义数据列表
data = []
# 遍历所有页面
for page in range(1, 101):
# 构造 URL
url = url_template.format(district, page, '/')
# 获取房源数据
house_data = get_house_data(url)
# 如果没有获取到数据,说明已经到达最后一页,退出循环
if not house_data:
break
# 将数据添加到列表中
data.extend(house_data)
# 将数据转换为 DataFrame 对象
df = pd.DataFrame(data, columns=['房源名称', '户型', '面向', '面积', '所属区', '地址', '价钱'])
# 添加时间列
df['时间'] = f'{start_year}-{end_year}'
return df
# 获取成都市锦江区2022年和2023年的所有房源数据
df1 = get_all_house_data('jinjiang', 2022, 2023)
# 获取成都市武侯区2022年和2023年的所有房源数据
df2 = get_all_house_data('wuhou', 2022, 2023)
# 将数据保存到 Excel 文件中
with pd.ExcelWriter('链家租房数据.xlsx') as writer:
df1.to_excel(writer, sheet_name='锦江区')
df2.to_excel(writer, sheet_name='武侯区')
```
相关推荐
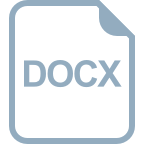














