帮我写一个java代码,能够解析小说txt文件,能够把章节名和章节内容分开,并将对应章节存储到chapter实体对象中,并将实体对象chapter加入list中
时间: 2023-12-23 13:04:46 浏览: 50
好的,以下是一个简单的Java代码,可以实现您的需求:
```java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
public class NovelParser {
public static void main(String[] args) {
// 定义章节列表
List<Chapter> chapterList = new ArrayList<>();
// 读取小说文件
File file = new File("novel.txt");
try (FileInputStream fis = new FileInputStream(file);
InputStreamReader isr = new InputStreamReader(fis, "UTF-8");
BufferedReader br = new BufferedReader(isr)) {
// 定义当前章节对象
Chapter currentChapter = null;
// 逐行读取小说文件
String line;
while ((line = br.readLine()) != null) {
// 判断当前行是否为章节名
if (line.matches("第.+章.+")) {
// 如果当前行为章节名,则将之前的章节对象加入章节列表中
if (currentChapter != null) {
chapterList.add(currentChapter);
}
// 创建新的章节对象
currentChapter = new Chapter(line, "");
} else {
// 如果当前行不是章节名,则将当前行的内容加入当前章节对象中
if (currentChapter != null) {
currentChapter.setContent(currentChapter.getContent() + line);
}
}
}
// 将最后一个章节对象加入章节列表中
if (currentChapter != null) {
chapterList.add(currentChapter);
}
} catch (Exception e) {
e.printStackTrace();
}
// 输出章节列表
for (Chapter chapter : chapterList) {
System.out.println(chapter.getTitle() + ":\n" + chapter.getContent() + "\n");
}
}
}
class Chapter {
private String title; // 章节名
private String content; // 章节内容
public Chapter(String title, String content) {
this.title = title;
this.content = content;
}
public String getTitle() {
return title;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
}
```
在这个代码中,我们首先定义了一个`Chapter`实体类,它有两个属性:`title`表示章节名,`content`表示章节内容。然后,我们通过`BufferedReader`类逐行读取小说文件,判断每一行是否为章节名,如果是,则创建新的`Chapter`对象,并将之前的`Chapter`对象加入章节列表中;如果不是,则将当前行的内容加入当前`Chapter`对象的内容中。最后,我们将所有的章节对象存储到`List`中,并输出每个章节的章节名和章节内容。
当然,这只是一个简单的示例代码,您可以根据自己的需求进行修改和完善。
相关推荐
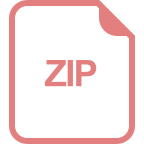
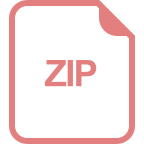
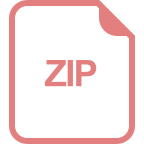














