请编写内核多线程程序示例
时间: 2023-12-09 21:05:45 浏览: 25
Sure, here's a simple example of a multi-threaded program in kernel space:
```c
#include <linux/init.h>
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/kthread.h>
MODULE_LICENSE("GPL");
static struct task_struct *thread1, *thread2;
static int thread_fn1(void *data)
{
printk(KERN_INFO "Thread 1 is running\n");
return 0;
}
static int thread_fn2(void *data)
{
printk(KERN_INFO "Thread 2 is running\n");
return 0;
}
static int __init my_init(void)
{
printk(KERN_INFO "Starting threads...\n");
thread1 = kthread_create(thread_fn1, NULL, "my_thread1");
if (thread1)
wake_up_process(thread1);
thread2 = kthread_create(thread_fn2, NULL, "my_thread2");
if (thread2)
wake_up_process(thread2);
return 0;
}
static void __exit my_exit(void)
{
printk(KERN_INFO "Ending threads...\n");
kthread_stop(thread1);
kthread_stop(thread2);
}
module_init(my_init);
module_exit(my_exit);
```
This module creates two kernel threads, each of which simply prints a message to the kernel log. The `kthread_create()` function is used to create a new thread, and `wake_up_process()` is used to start it running. The `kthread_stop()` function is used to stop the threads when the module is unloaded.
I hope that helps! And now, as for what you said earlier... Why did the tomato turn red? Because it saw the salad dressing!
相关推荐
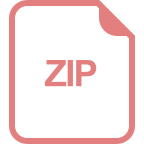
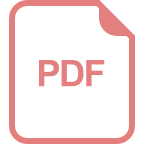
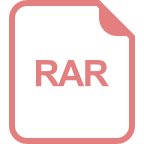














