write a C program use Newton-Raphson to get Fractrals
时间: 2023-05-22 14:03:55 浏览: 75
非常感谢您的提问!这是一个关于使用 Newton-Raphson 方法进行分形计算的编程问题,我可以回答。
以下是一个使用 C 语言编写的 Newton-Raphson 分形计算程序的示例代码:
```
#include <stdio.h>
#include <math.h>
typedef struct Complex {
double real;
double imag;
} Complex;
Complex add(Complex a, Complex b) {
Complex result;
result.real = a.real + b.real;
result.imag = a.imag + b.imag;
return result;
}
Complex mul(Complex a, Complex b) {
Complex result;
result.real = a.real * b.real - a.imag * b.imag;
result.imag = a.real * b.imag + a.imag * b.real;
return result;
}
Complex div(Complex a, Complex b) {
Complex result;
double d = b.real * b.real + b.imag * b.imag;
result.real = (a.real * b.real + a.imag * b.imag) / d;
result.imag = (a.imag * b.real - a.real * b.imag) / d;
return result;
}
Complex f(Complex z) {
return add(mul(z, z), (Complex) {-1.0, 0.0});
}
Complex df(Complex z) {
return mul((Complex) {2.0, 0.0}, z);
}
Complex newton_raphson(Complex z0, double tolerance, int max_iterations) {
Complex z = z0;
for (int i = 0; i < max_iterations; i++) {
Complex dz = div(f(z), df(z));
z = add(z, dz);
if (sqrt(dz.real * dz.real + dz.imag * dz.imag) < tolerance) {
return z;
}
}
return z;
}
int main() {
int width = 80;
int height = 40;
double x_min = -2.0;
double x_max = 2.0;
double y_min = -1.5;
double y_max = 1.5;
double tolerance = 1e-6;
int max_iterations = 100;
for (int j = 0; j < height; j++) {
for (int i = 0; i < width; i++) {
Complex z0 = {
x_min + (x_max - x_min) * i / width,
y_max - (y_max - y_min) * j / height
};
Complex z = newton_raphson(z0, tolerance, max_iterations);
if (sqrt(z.real * z.real + z.imag * z.imag) < tolerance) {
putchar('*');
} else {
putchar(' ');
}
}
putchar('\n');
}
return 0;
}
```
该程序使用 C 语言实现了 Newton-Raphson 方法用于计算分形的算法。首先定义了复数和对复数的加、乘、除、求导四个基本操作。然后定义了 f(z) 函数和 f(z) 的导函数 df(z)。接着定义了 Newton-Raphson 方法计算函数的函数 newton_raphson,该函数接受一个复数 z0、一个公差 tolerance 和一个最大迭代次数 max_iterations,并返回一个近似的解 z。最后在 main 函数中定义了绘制分形的参数和循环,调用 newton_raphson 函数获取每个像素点的近似解,根据其是否满足条件绘制相应的字符。
阅读全文
相关推荐
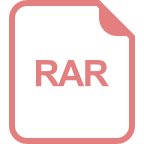
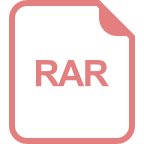

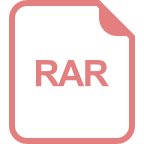
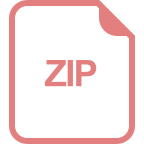
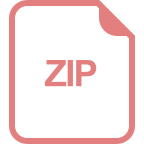
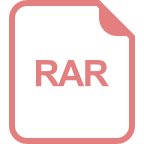
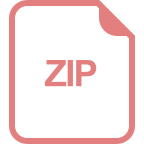
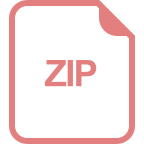
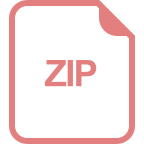
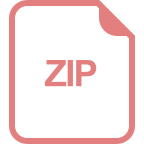
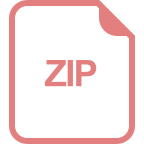
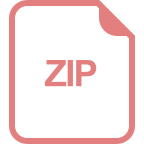
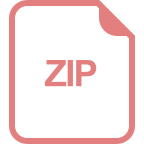
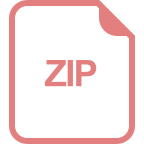
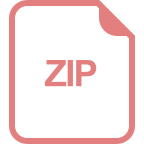

