用spring boot编写一个个人博客系统中的标签/分类管理功能
时间: 2024-05-01 14:19:48 浏览: 23
好的,下面是用 Spring Boot 编写个人博客系统中的标签/分类管理功能的步骤:
1. 首先,需要创建一个 Tag(标签)类和一个 Category(分类)类。它们可以包含以下字段:
```
public class Tag {
private Long id;
private String name;
// getter and setter methods
}
public class Category {
private Long id;
private String name;
// getter and setter methods
}
```
2. 接下来,创建一个 TagService 和一个 CategoryService 类来处理标签和分类的业务逻辑。它们可以包含以下方法:
```
public interface TagService {
List<Tag> findAllTags();
Tag findTagById(Long id);
void saveTag(Tag tag);
void deleteTag(Long id);
}
public interface CategoryService {
List<Category> findAllCategories();
Category findCategoryById(Long id);
void saveCategory(Category category);
void deleteCategory(Long id);
}
```
3. 然后,创建一个 TagController 和一个 CategoryController 类来处理与标签和分类相关的 HTTP 请求。它们可以包含以下方法:
```
@RestController
@RequestMapping("/api/tags")
public class TagController {
@Autowired
private TagService tagService;
@GetMapping("/")
public List<Tag> getAllTags() {
return tagService.findAllTags();
}
@GetMapping("/{id}")
public Tag getTagById(@PathVariable Long id) {
return tagService.findTagById(id);
}
@PostMapping("/")
public void addTag(@RequestBody Tag tag) {
tagService.saveTag(tag);
}
@DeleteMapping("/{id}")
public void deleteTag(@PathVariable Long id) {
tagService.deleteTag(id);
}
}
@RestController
@RequestMapping("/api/categories")
public class CategoryController {
@Autowired
private CategoryService categoryService;
@GetMapping("/")
public List<Category> getAllCategories() {
return categoryService.findAllCategories();
}
@GetMapping("/{id}")
public Category getCategoryById(@PathVariable Long id) {
return categoryService.findCategoryById(id);
}
@PostMapping("/")
public void addCategory(@RequestBody Category category) {
categoryService.saveCategory(category);
}
@DeleteMapping("/{id}")
public void deleteCategory(@PathVariable Long id) {
categoryService.deleteCategory(id);
}
}
```
4. 最后,在应用程序的启动类中,添加注解 @EnableWebMvc 和 @ComponentScan,并配置视图解析器和静态资源路径:
```
@SpringBootApplication
@EnableWebMvc
@ComponentScan(basePackages = {"com.example.blog"})
public class BlogApplication implements WebMvcConfigurer {
// configure view resolver
@Bean
public ViewResolver viewResolver() {
InternalResourceViewResolver resolver = new InternalResourceViewResolver();
resolver.setPrefix("/WEB-INF/views/");
resolver.setSuffix(".html");
return resolver;
}
// configure static resources
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/static/**").addResourceLocations("classpath:/static/");
}
public static void main(String[] args) {
SpringApplication.run(BlogApplication.class, args);
}
}
```
这样就完成了个人博客系统中的标签/分类管理功能的编写。
相关推荐
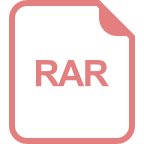
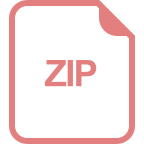
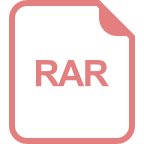














