用java代码写一个http请求
时间: 2023-09-10 10:05:39 浏览: 107
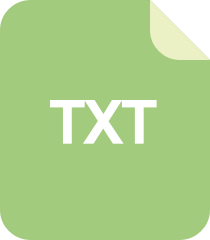
关于http请求的相关java代码
### 回答1:
我可以给你一个简单的Java代码示例:URL url = new URL("http://www.example.com/");
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
### 回答2:
下面是一个使用Java代码编写HTTP请求的示例:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpRequestExample {
public static void main(String[] args) {
String url = "https://www.example.com"; // 要发送请求的URL
try {
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET"); // 设置请求方法为GET
int responseCode = con.getResponseCode(); // 获取响应码
System.out.println("响应码: " + responseCode);
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println("响应内容: " + response.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这是一个简单的使用URL和HttpURLConnection类的示例。它发送一个GET请求,并输出响应的状态码和内容。你可以根据实际需求进行修改和扩展,例如添加请求头,发送POST请求等等。
### 回答3:
在Java中进行HTTP请求可以使用各种库,例如原生的HttpURLConnection、Apache的HttpClient等。以下是一个使用HttpURLConnection发送GET请求的示例代码:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpRequestExample {
public static void main(String[] args) {
String urlStr = "http://example.com";
try {
// 创建URL对象
URL url = new URL(urlStr);
// 打开连接
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 设置请求方法为GET
connection.setRequestMethod("GET");
// 发送请求
int responseCode = connection.getResponseCode();
// 处理响应
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
System.out.println(response.toString());
} else {
System.out.println("HTTP请求失败,响应码:" + responseCode);
}
// 关闭连接
connection.disconnect();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
上述代码首先创建一个URL对象,传入待请求的URL地址。然后通过打开连接的方式创建一个HttpURLConnection对象,并设置请求方法为GET。通过调用`getResponseCode()`方法获取响应码,如果响应码为200表示请求成功,可以通过`getInputStream()`方法获取响应的输入流,并通过读取输入流的方式获取响应内容。最后,关闭连接。
需要注意的是,为了处理待请求的URL地址以及处理请求结果的方式,可能需要使用try-catch来捕获可能抛出的异常来处理错误情况。
阅读全文
相关推荐
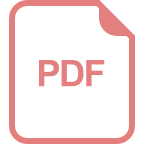
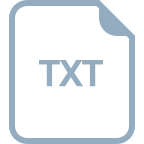
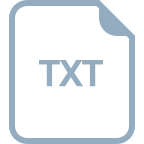
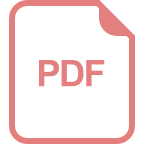
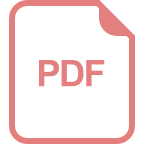
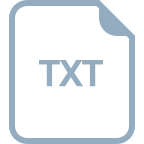
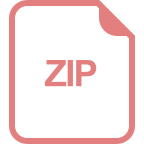
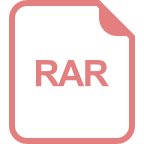
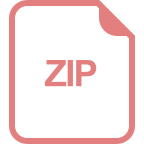
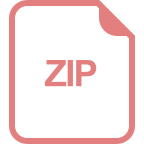
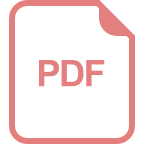
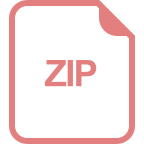