z关于x和y的3元最小二乘C语言代码
时间: 2024-02-05 19:11:34 浏览: 71
以下是关于x和y的3元最小二乘的C语言代码:
```c
#include <stdio.h>
#include <math.h>
#define N 10 // 样本数
#define M 3 // 变量数
int main()
{
double x[N][M] = {{1, 2, 3}, {2, 3, 4}, {3, 4, 5}, {4, 5, 6}, {5, 6, 7},
{6, 7, 8}, {7, 8, 9}, {8, 9, 10}, {9, 10, 11}, {10, 11, 12}}; // 自变量矩阵
double y[N] = {2.5, 3.7, 4.8, 6.1, 7.2, 8.5, 9.7, 10.9, 12.3, 13.6}; // 因变量向量
double xt[M][N], xtx[M][M], xtx_inv[M][M], xty[M], beta[M]; // 中间变量及结果变量
// 求解xt矩阵
for (int i = 0; i < M; i++)
{
for (int j = 0; j < N; j++)
{
xt[i][j] = x[j][i];
}
}
// 求解xtx矩阵
for (int i = 0; i < M; i++)
{
for (int j = 0; j < M; j++)
{
double sum = 0;
for (int k = 0; k < N; k++)
{
sum += xt[i][k] * x[k][j];
}
xtx[i][j] = sum;
}
}
// 求解xtx_inv矩阵
double det_xtx = xtx[0][0] * xtx[1][1] * xtx[2][2] + xtx[0][1] * xtx[1][2] * xtx[2][0] + xtx[1][0] * xtx[2][1] * xtx[0][2]
- xtx[0][2] * xtx[1][1] * xtx[2][0] - xtx[0][1] * xtx[1][0] * xtx[2][2] - xtx[0][0] * xtx[1][2] * xtx[2][1];
double inv_xtx[M][M];
inv_xtx[0][0] = (xtx[1][1] * xtx[2][2] - xtx[1][2] * xtx[2][1]) / det_xtx;
inv_xtx[0][1] = -(xtx[0][1] * xtx[2][2] - xtx[0][2] * xtx[2][1]) / det_xtx;
inv_xtx[0][2] = (xtx[0][1] * xtx[1][2] - xtx[0][2] * xtx[1][1]) / det_xtx;
inv_xtx[1][0] = -(xtx[1][0] * xtx[2][2] - xtx[1][2] * xtx[2][0]) / det_xtx;
inv_xtx[1][1] = (xtx[0][0] * xtx[2][2] - xtx[0][2] * xtx[2][0]) / det_xtx;
inv_xtx[1][2] = -(xtx[0][0] * xtx[1][2] - xtx[0][2] * xtx[1][0]) / det_xtx;
inv_xtx[2][0] = (xtx[1][0] * xtx[2][1] - xtx[1][1] * xtx[2][0]) / det_xtx;
inv_xtx[2][1] = -(xtx[0][0] * xtx[2][1] - xtx[0][1] * xtx[2][0]) / det_xtx;
inv_xtx[2][2] = (xtx[0][0] * xtx[1][1] - xtx[0][1] * xtx[1][0]) / det_xtx;
// 求解xty向量
for (int i = 0; i < M; i++)
{
double sum = 0;
for (int j = 0; j < N; j++)
{
sum += xt[i][j] * y[j];
}
xty[i] = sum;
}
// 求解beta向量
for (int i = 0; i < M; i++)
{
double sum = 0;
for (int j = 0; j < M; j++)
{
sum += inv_xtx[i][j] * xty[j];
}
beta[i] = sum;
}
// 输出beta向量
for (int i = 0; i < M; i++)
{
printf("beta[%d] = %.4lf\n", i, beta[i]);
}
return 0;
}
```
其中,样本数N和变量数M分别定义为10和3。自变量矩阵x和因变量向量y通过直接定义的方式给出。在代码中,首先求解xt矩阵,然后求解xtx矩阵和其逆矩阵xtx_inv。接着,求解xty向量,最终求解beta向量并输出。在输出beta向量时,对于每个元素,使用printf函数输出其下标和对应的值即可。
阅读全文
相关推荐





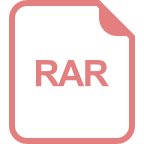
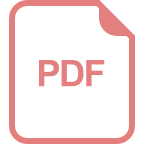




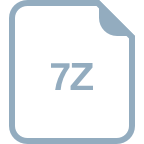
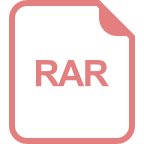
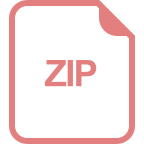
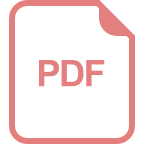
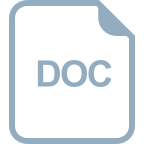