用Java创建两个方法作为乌龟和兔子的线程,在main方法中调用两种方法实现龟兔赛跑
时间: 2023-05-25 13:02:47 浏览: 145
由于这个问题没有明确具体要求,所以提供一种可能的解决方案:
```java
public class TurtleAndRabbitRace {
public static void main(String[] args) {
Thread turtleThread = new Thread(() -> turtleRace());
Thread rabbitThread = new Thread(() -> rabbitRace());
turtleThread.start();
rabbitThread.start();
try {
turtleThread.join();
rabbitThread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public static void turtleRace() {
int totalDistance = 100;
int turtlePosition = 0;
while (turtlePosition < totalDistance) {
System.out.println("Turtle is at " + turtlePosition + "...");
turtlePosition += (int) (Math.random() * 10);
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("Turtle wins!");
}
public static void rabbitRace() {
int totalDistance = 100;
int rabbitPosition = 0;
while (rabbitPosition < totalDistance) {
System.out.println("Rabbit is at " + rabbitPosition + "...");
if (Math.random() < 0.3) {
// Rabbit takes a nap
System.out.println("Rabbit takes a nap...");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
rabbitPosition += (int) (Math.random() * 20);
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("Rabbit wins!");
}
}
```
这个程序通过创建两个线程,一个模拟乌龟的线程,一个模拟兔子的线程,让它们并行地进行赛跑。在每个线程中,都有一个循环用来模拟赛跑的过程。乌龟的线程每次前进的距离是随机的(最多为10),兔子的线程每次前进的距离也是随机的(最多为20)。但是兔子有时会犯困,这时它会在路边小憩一会儿,让乌龟有机会赶上来。具体来说,每次兔子前进时,有30%的概率它会停下来休息1秒钟。
当某个线程达到了终点(距离为100)时,它会输出一条获胜的消息。程序在main方法中启动两个线程,并等待它们都完成后才退出。
阅读全文
相关推荐
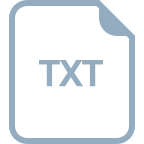
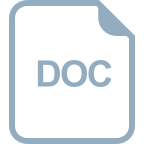
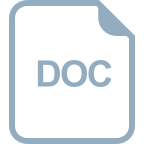












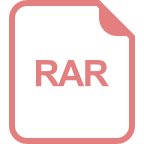
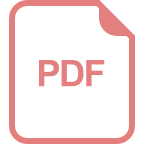