用c++设计一个链表类
时间: 2023-10-31 08:04:34 浏览: 46
以下是一个简单的链表类的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构体
typedef struct node {
int data;
struct node *next;
} Node;
// 链表类
typedef struct linkedList {
Node *head; // 链表头节点
int size; // 链表大小
} LinkedList;
// 初始化链表
void initLinkedList(LinkedList *list) {
list->head = NULL;
list->size = 0;
}
// 判断链表是否为空
int isEmpty(LinkedList *list) {
return list->size == 0;
}
// 获取链表大小
int getSize(LinkedList *list) {
return list->size;
}
// 在链表头添加节点
void addFirst(LinkedList *list, int data) {
Node *newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = list->head;
list->head = newNode;
list->size++;
}
// 在链表末尾添加节点
void addLast(LinkedList *list, int data) {
Node *newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
if (isEmpty(list)) {
list->head = newNode;
} else {
Node *cur = list->head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = newNode;
}
list->size++;
}
// 删除链表头节点
void removeFirst(LinkedList *list) {
if (!isEmpty(list)) {
Node *temp = list->head;
list->head = temp->next;
free(temp);
list->size--;
}
}
// 删除链表末尾节点
void removeLast(LinkedList *list) {
if (!isEmpty(list)) {
if (list->size == 1) {
free(list->head);
list->head = NULL;
} else {
Node *cur = list->head;
while (cur->next->next != NULL) {
cur = cur->next;
}
free(cur->next);
cur->next = NULL;
}
list->size--;
}
}
// 打印链表
void printList(LinkedList *list) {
if (isEmpty(list)) {
printf("List is empty.\n");
} else {
Node *cur = list->head;
while (cur != NULL) {
printf("%d ", cur->data);
cur = cur->next;
}
printf("\n");
}
}
int main() {
LinkedList list;
initLinkedList(&list);
addFirst(&list, 1);
addFirst(&list, 2);
addLast(&list, 3);
printList(&list);
removeFirst(&list);
removeLast(&list);
printList(&list);
return 0;
}
```
以上代码实现了链表的基本操作,包括初始化链表、判断链表是否为空、获取链表大小、在链表头和末尾添加节点、删除链表头和末尾节点、打印链表等。可以根据需要进行扩展,例如在指定位置插入节点、查找节点、反转链表等。
相关推荐
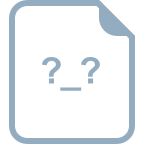
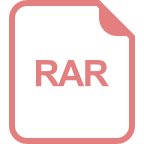
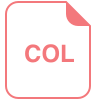
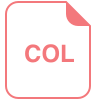












