int alphaBeta(int depth, int alpha, int beta, int& startX, int& startY, int& resultX, int& resultY) { if (depth == MAX_DEPTH || checkWin(startX, startY, currBotColor) || checkWin(startX, startY, -currBotColor)) return evaluate(); int color = depth % 2 == 0 ? currBotColor : -currBotColor; int bestScore = color == currBotColor ? -INF : INF; for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { if (gridInfo[i][j] != grid_blank) continue; gridInfo[i][j] = color; int score = 0; if (depth == 0) { startX = i; startY = j; } int tmpX, tmpY; score = alphaBeta(depth + 1, alpha, beta, startX, startY, tmpX, tmpY); gridInfo[i][j] = grid_blank; if (color == currBotColor) { if (score > bestScore) { bestScore = score; if (depth == 0) { resultX = i; resultY = j; } } alpha = max(alpha, bestScore); } else { if (score < bestScore) { bestScore = score; if (depth == 0) { resultX = i; resultY = j; } } beta = min(beta, bestScore); } if (beta <= alpha) break; } } return bestScore; } 这个代码为什么要返回bestScore
时间: 2024-02-10 07:08:39 浏览: 52
这个代码是一个alpha-beta剪枝算法的实现,返回的`bestScore`表示当前搜索路径下的最优得分,也就是当前搜索到的局面下,当前玩家能够获得的最大收益或者最小损失。
在这个算法中,我们要求的是当前玩家在搜索路径中能够获得的最大收益或者最小损失,因此我们需要用`bestScore`来记录当前搜索路径下的最优得分。
在搜索过程中,我们会不断更新`bestScore`的值,并且根据当前搜索深度的奇偶性来决定是极小节点还是极大节点,从而更新`alpha`或者`beta`的值,以进行剪枝。最终,当搜索深度达到最大深度,或者搜索到了终局状态,我们就可以返回`bestScore`,表示当前搜索路径下的最优得分。
需要注意的是,在搜索过程中,我们只更新当前搜索路径下的最优得分,而不是整个搜索空间下的最优得分。因此,我们需要在每个搜索路径中维护一个`bestScore`,而不是在整个搜索空间中维护一个全局的`bestScore`。
相关问题
逐行详细解释以下代码,包括每个参数的意义:int alphaBeta(int depth, int alpha, int beta, int& startX, int& startY, int& resultX, int& resultY) { if (depth == MAX_DEPTH || checkWin(startX, startY, currBotColor) || checkWin(startX, startY, -currBotColor)) return evaluate(); int color = depth % 2 == 0 ? currBotColor : -currBotColor; int bestScore = color == currBotColor ? -INF : INF; for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { if (gridInfo[i][j] != grid_blank) continue; gridInfo[i][j] = color; int score = 0; if (depth == 0) { startX = i; startY = j; } int tmpX, tmpY; score = alphaBeta(depth + 1, alpha, beta, startX, startY, tmpX, tmpY); gridInfo[i][j] = grid_blank; if (color == currBotColor) { if (score > bestScore) { bestScore = score; if (depth == 0) { resultX = i; resultY = j; } } alpha = max(alpha, bestScore); } else { if (score < bestScore) { bestScore = score; if (depth == 0) { resultX = i; resultY = j; } } beta = min(beta, bestScore); } if (beta <= alpha) break; } } return bestScore; }
这段代码实现了一个alpha-beta剪枝算法,用于搜索最优解的过程中进行剪枝,以提高搜索效率。下面是代码的逐行解释:
1. int alphaBeta(int depth, int alpha, int beta, int& startX, int& startY, int& resultX, int& resultY)
定义了一个函数 alphaBeta,它有6个参数,分别是搜索深度depth、alpha、beta和起始坐标startX、startY,以及最终结果坐标resultX、resultY。
2. if (depth == MAX_DEPTH || checkWin(startX, startY, currBotColor) || checkWin(startX, startY, -currBotColor)) return evaluate();
如果搜索深度等于预设的最大深度MAX_DEPTH,或者起始坐标已经胜利了,就直接返回当前状态的评估值evaluate()。
3. int color = depth % 2 == 0 ? currBotColor : -currBotColor;
定义变量color表示当前的颜色。
4. int bestScore = color == currBotColor ? -INF : INF;
定义变量bestScore表示当前的最优分数。如果当前颜色为机器人的颜色currBotColor,则初始化为负无穷-INF,否则初始化为正无穷INF。
5. for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) {
两个循环遍历棋盘上的所有格子。
6. if (gridInfo[i][j] != grid_blank) continue;
如果当前格子不为空,则跳过该格子。
7. gridInfo[i][j] = color;
将当前格子填上当前颜色color。
8. int score = 0;
初始化分数为0。
9. if (depth == 0) { startX = i; startY = j; }
如果搜索深度为0,即开始搜索的时候,记录下当前的起始坐标startX和startY。
10. int tmpX, tmpY; score = alphaBeta(depth + 1, alpha, beta, startX, startY, tmpX, tmpY);
递归搜索下一层,将搜索深度加1,alpha和beta值不变,起始坐标为startX和startY,最终结果坐标为tmpX和tmpY,返回搜索结果的分数score。
11. gridInfo[i][j] = grid_blank;
恢复当前格子为空。
12. if (color == currBotColor) { if (score > bestScore) { bestScore = score; if (depth == 0) { resultX = i; resultY = j; } } alpha = max(alpha, bestScore); } else { if (score < bestScore) { bestScore = score; if (depth == 0) { resultX = i; resultY = j; } } beta = min(beta, bestScore); }
根据当前搜索的颜色color和机器人的颜色currBotColor进行判断,更新最优分数bestScore,如果当前深度为0,更新最终结果坐标resultX和resultY,同时更新alpha和beta的值。
13. if (beta <= alpha) break;
进行alpha-beta剪枝,如果beta小于等于alpha,就直接退出循环。
14. return bestScore;
返回当前最优分数bestScore。
int alphaBeta(int depth, int alpha, int beta, int& startX, int& startY, int& resultX, int& resultY) { if (depth == MAX_DEPTH || checkWin(startX, startY, currBotColor) || checkWin(startX, startY, -currBotColor)) return evaluate(); int color = depth % 2 == 0 ? currBotColor : -currBotColor; int bestScore = color == currBotColor ? -INF : INF; for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { if (gridInfo[i][j] != grid_blank) continue; gridInfo[i][j] = color; int score = 0; if (depth == 0) { startX = i; startY = j; } int tmpX, tmpY; score = alphaBeta(depth + 1, alpha, beta, startX, startY, tmpX, tmpY); gridInfo[i][j] = grid_blank; if (color == currBotColor) { if (score > bestScore) { bestScore = score; if (depth == 0) { resultX = i; resultY = j; } } alpha = max(alpha, bestScore); } else { if (score < bestScore) { bestScore = score; if (depth == 0) { resultX = i; resultY = j; } } beta = min(beta, bestScore); } if (beta <= alpha) break; } } return bestScore; } 用这个代码的话主函数还需要什么,才能得到六子棋当前局决策的两步棋的坐标
这个代码实现了一个alpha-beta剪枝算法,用于搜索六子棋的最优决策。
如果要使用这个算法来得到当前局面的最优决策,你需要在主函数中调用这个算法,并传入当前的棋盘状态和当前玩家的颜色。具体来说,你需要进行如下操作:
1. 定义变量`startX`、`startY`、`resultX`和`resultY`,用于存储当前局面的初始位置以及最优决策的位置。
2. 调用`alphaBeta`函数,传入当前的搜索深度、负无穷大和正无穷大作为`alpha`和`beta`的初值,以及定义好的`startX`、`startY`、`resultX`和`resultY`。
3. 在`alphaBeta`函数返回之后,`resultX`和`resultY`就是当前局面的最优决策的坐标。
需要注意的是,在调用`alphaBeta`函数之前,你需要保证当前棋盘上至少有一个空位,否则算法无法进行。此外,你还需要定义好`checkWin`函数和`evaluate`函数,分别用于判断游戏是否结束和评估当前局面的得分。
阅读全文
相关推荐
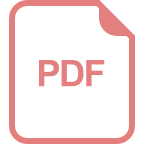
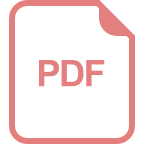
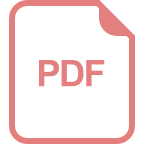














