使用python为excel添加一个空的sheet2,sheet1不变
时间: 2024-01-23 12:04:16 浏览: 27
可以使用openpyxl库来实现为excel添加一个空的sheet2,同时保留sheet1不变,代码如下:
```python
import openpyxl
# 打开excel文件
wb = openpyxl.load_workbook('example.xlsx')
# 创建新的sheet
wb.create_sheet('sheet2')
# 选中sheet1
ws1 = wb['Sheet1']
# 将新的sheet2插入到sheet1之后
wb.move_sheet('sheet2', offset=ws1.index)
# 保存文件
wb.save('example.xlsx')
```
注意:需要确保在执行上述代码前已经安装了openpyxl库。
相关问题
使用python保持sheet1不变,添加一个空的sheet2
你可以使用 Python 中的 openpyxl 库来实现这个操作。下面是一个示例代码:
```python
from openpyxl import Workbook
# 创建一个新的 Excel 文件对象
workbook = Workbook()
# 获取默认的 worksheet(sheet1)
worksheet = workbook.active
# 创建一个新的 worksheet(sheet2)
worksheet2 = workbook.create_sheet(title="Sheet2")
# 保存文件
workbook.save('example.xlsx')
```
在这个示例中,我们首先使用 Workbook() 函数创建一个新的 Excel 文件对象。默认情况下,该对象会包含一个工作表(worksheet),也就是 sheet1。然后,我们使用 create_sheet() 函数创建一个新的工作表,命名为 Sheet2。最后,我们使用 save() 函数将所有的更改保存到一个名为 example.xlsx 的文件中。
这样,你就可以在保持 sheet1 不变的同时,添加一个名为 Sheet2 的空工作表了。
python中将df写入到一个sheet,其他sheet内容不变
可以使用 `openpyxl` 库来实现将 DataFrame 写入到指定的 Excel 文件的指定 Sheet 中,同时保留其他 Sheet 的内容。
首先,需要安装 `openpyxl` 库,可以使用 pip 进行安装:
```python
pip install openpyxl
```
接下来,假设要将 DataFrame 写入到 `example.xlsx` 文件的 `Sheet2` 中,可以按照以下步骤实现:
1. 导入需要的库
```python
import pandas as pd
from openpyxl import load_workbook
```
2. 读取 Excel 文件
```python
# 读取 Excel 文件
book = load_workbook('example.xlsx')
```
3. 获取 Sheet 对象
```python
# 获取 Sheet 对象
writer = pd.ExcelWriter('example.xlsx', engine='openpyxl')
writer.book = book
writer.sheets = dict((ws.title, ws) for ws in book.worksheets)
sheetname = 'Sheet2'
ws = writer.sheets[sheetname]
```
4. 将 DataFrame 写入到指定 Sheet
```python
# 将 DataFrame 写入到指定 Sheet
df.to_excel(writer, sheet_name=sheetname, index=False, header=False, startrow=ws.max_row+1)
```
最后,记得关闭 writer 对象:
```python
writer.save()
writer.close()
```
完整代码如下:
```python
import pandas as pd
from openpyxl import load_workbook
# 读取 Excel 文件
book = load_workbook('example.xlsx')
# 获取 Sheet 对象
writer = pd.ExcelWriter('example.xlsx', engine='openpyxl')
writer.book = book
writer.sheets = dict((ws.title, ws) for ws in book.worksheets)
sheetname = 'Sheet2'
ws = writer.sheets[sheetname]
# 将 DataFrame 写入到指定 Sheet
df.to_excel(writer, sheet_name=sheetname, index=False, header=False, startrow=ws.max_row+1)
# 保存并关闭 writer 对象
writer.save()
writer.close()
```
相关推荐
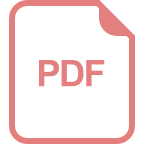
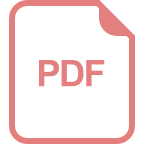
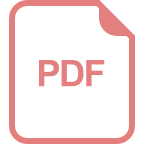












