具体解释void changeScores() //成绩修改 { printf("Enter password:"); //输入密码 char pwd[PROFILE_LENGTH]; scanf("%s", pwd); //输入密码 if (compare(password, pwd) != 0) { printf("密码错误!\n"); return; } printf("Enter student id:"); char id[PROFILE_LENGTH]; scanf("%s", id); //输入学生学号 int i = studentCount; for (i = 0; i < studentCount; i++) { if (compare(students[i].id, id) == 0) break; } if (i == studentCount) { printf("No student %s\n", id); return; } printf("Courses: "); for (int j = 0; j < courseCount; j++) //输出课程名字 { printf(" %s", courses[j].name); } printf("\nEnter the scores:"); for (int j = 0; j < courseCount; j++) //输入更改后的成绩 { scanf("%d", &students[i].scores[j]); } }
时间: 2024-04-02 10:33:25 浏览: 94
这段代码实现了一个名为 `changeScores` 的函数,用于修改学生的成绩。具体实现如下:
首先,该函数会要求用户输入一个密码,如果密码输入错误则直接返回。接着,用户需要输入一个学生的学号,程序会在学生数组中查找该学号对应的学生,如果找不到则返回。如果找到了该学生,则程序会输出所有课程的名称,然后用户需要输入修改后的成绩。程序会依次接收用户输入的成绩,并将其更新到该学生的成绩数组中。
值得注意的是,该函数中使用了一些之前实现的辅助函数,比如 `compare` 函数用于比较密码和学生学号是否匹配。此外,该函数的实现也依赖于一些全局变量,比如 `studentCount` 表示学生数组的长度,`courseCount` 表示课程数组的长度,`students` 和 `courses` 分别表示学生和课程数组。
相关问题
具体解释void changeScores() //成绩修改 { printf("Enter password:"); //输入密码 char pwd[PROFILE_LENGTH]; scanf("%s", pwd); //输入密码 if (compare(password, pwd) != 0) { printf("密码错误!\n"); return; } printf("Enter student id:"); char id[PROFILE_LENGTH]; scanf("%s", id); //输入学生学号 int i = studentCount; for (i = 0; i < studentCount; i++) { if (compare(students[i].id, id) == 0) break; } if (i == studentCount) { printf("No student %s\n", id); return; } printf("Courses: "); for (int j = 0; j < courseCount; j++) //输出课程名字 { printf(" %s", courses[j].name); } printf("\nEnter the scores:"); for (int j = 0; j < courseCount; j++) //输入更改后的成绩 { scanf("%d", &students[i].scores[j]); } }
以上代码是一个 C 语言函数,函数名为 changeScores,用于修改学生的成绩。该函数需要输入密码和学生学号,并且只有在密码正确且学生存在的情况下才能进行成绩的修改。在函数中,通过遍历学生数组查找输入的学生学号是否存在,如果不存在则提示错误信息并返回。如果存在,则输出课程名字,并且连续输入需要修改的成绩,最后将修改后的成绩存储在学生数组中。该函数有效地保护了学生信息的安全,并且实现了课程成绩的修改功能。
#include <stdio.h> #include <stdlib.h> #include <string.h> #define BLUE "\033[34m" #define CYAN "\033[36m" #define GREEN "\033[32m" #define RED "\033[31m" #define RESET "\033[0m" #define YELLOW "\033[33m" const char* logo = " ____ __\n / __ )____ _/ /_____ _\n / __ / __ `/ __/ __ `/ \n / /_/ / /_/ / /_/ /_/ / \n/_____/\\__,_/\\__/\\__,_/ \n"; const char* welcome = "欢迎使用模拟 QQ 登录界面\n"; const char* tip_user = "请输入用户名:"; const char* tip_pwd = "请输入密码:"; const char* success = "登录成功!\n"; const char* failure = "用户名或密码错误,登录失败!\n"; int main() { char username[20], password[20], buffer[256]; printf(CYAN "%s" RESET, logo); printf(BLUE "%s" RESET, welcome); printf(YELLOW "%s" RESET, tip_user); fgets(buffer, sizeof(buffer), stdin); sscanf(buffer, "%s", username); printf(YELLOW "%s" RESET, tip_pwd); fgets(buffer, sizeof(buffer), stdin); sscanf(buffer, "%s", password); if (strcmp(username, "qquser") == 0 && strcmp(password, "qqpassword") == 0) { printf(GREEN "%s" RESET, success); } else { printf(RED "%s" RESET, failure); } return 0; }
这段代码是一个模拟 QQ 登录界面的程序,使用了 C 语言编写。它会先输出一个 QQ 的 Logo,然后打印欢迎信息和提示用户输入用户名和密码。接着,使用 fgets() 函数从标准输入中读取用户输入的字符串,并使用 sscanf() 函数将其存储到相应的变量中。最后,通过 strcmp() 函数比较用户名和密码是否正确,如果输入正确则输出登录成功的信息,否则输出登录失败的信息。程序中使用了 ANSI Escape Code 来控制输出文本的颜色,让界面看起来更加美观。
阅读全文
相关推荐
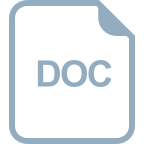
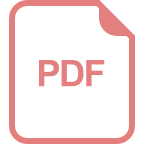
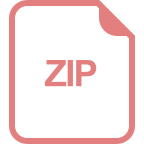


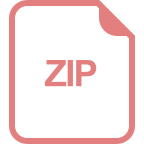
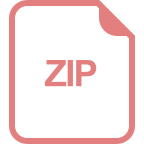
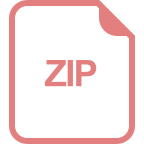
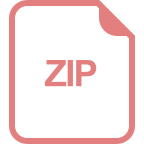
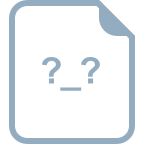
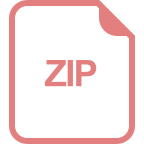
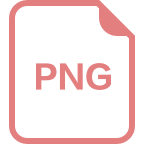
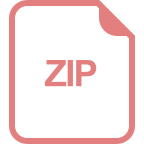
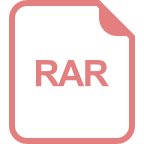
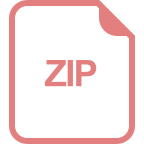
