ValueError: Input 0 of layer conv2d is incompatible with the layer: : expected min_ndim=4, found ndim=3. Full shape received: (None, 1024, 1024).
时间: 2023-11-27 11:48:58 浏览: 159
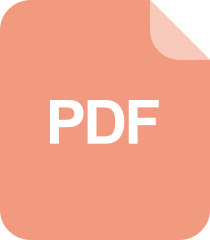
Python 3.x 新特性及10大变化
这个错误通常是因为输入的张量维度不是 4 维的。Conv2D 层期望输入的张量至少是 4 维的,其中前三维分别是样本数、高度和宽度,最后一维是通道数。但是,你提供的输入张量只有 3 维,形状为 `(None, 1024, 1024)`,缺少了最后一个维度的信息。
你需要将输入张量的形状转换为 4 维,可以通过 `tf.expand_dims()` 函数来增加一个维度来实现。例如,如果你的数据是灰度图像,可以使用以下代码将其转换为 4 维张量:
```
import tensorflow as tf
# x 是 3 维张量,形状为 (None, 1024, 1024)
x = tf.placeholder(tf.float32, shape=[None, 1024, 1024])
# 将 x 转换为 4 维张量,形状为 (None, 1024, 1024, 1)
x = tf.expand_dims(x, axis=-1)
```
如果你的数据是彩色图像,可以将最后一个维度的大小设置为 3,表示 R、G、B 三个通道:
```
import tensorflow as tf
# x 是 3 维张量,形状为 (None, 1024, 1024, 3)
x = tf.placeholder(tf.float32, shape=[None, 1024, 1024, 3])
```
这样,你就可以将转换后的张量输入到 Conv2D 层中了。
阅读全文
相关推荐
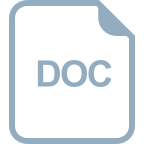
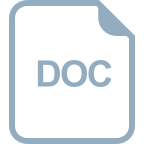















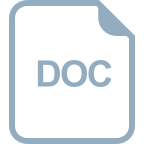