请使用c++语言编写可以计算二叉树的节点总数和深度的代码
时间: 2023-06-14 17:03:56 浏览: 102
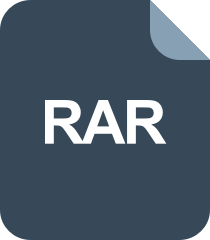
数据结构实验代码求树的节点总数.rar
下面是使用C语言实现计算二叉树节点总数和深度的代码:
```
#include <stdio.h>
#include <stdlib.h>
//定义二叉树结构体
typedef struct Node {
int value; //节点值
struct Node* left; //左子树指针
struct Node* right; //右子树指针
} Node;
//创建节点函数
Node* createNode(int value) {
Node* node = (Node*) malloc(sizeof(Node));
node->value = value;
node->left = NULL;
node->right = NULL;
return node;
}
//计算二叉树节点总数
int countNodes(Node* root) {
if (root == NULL) {
return 0;
}
return 1 + countNodes(root->left) + countNodes(root->right);
}
//计算二叉树深度
int treeDepth(Node* root) {
if (root == NULL) {
return 0;
}
int leftDepth = treeDepth(root->left);
int rightDepth = treeDepth(root->right);
return (leftDepth > rightDepth) ? (leftDepth + 1) : (rightDepth + 1);
}
int main() {
//创建二叉树
Node* root = createNode(1);
root->left = createNode(2);
root->right = createNode(3);
root->left->left = createNode(4);
root->left->right = createNode(5);
root->right->left = createNode(6);
root->right->right = createNode(7);
//计算节点总数和深度
int nodeCount = countNodes(root);
int treeDepth = treeDepth(root);
//输出结果
printf("节点总数:%d\n", nodeCount);
printf("深度:%d\n", treeDepth);
return 0;
}
```
这段代码中,我们使用了递归的方式来计算二叉树的节点总数和深度。函数`countNodes`和`treeDepth`分别用于计算节点总数和深度。在`main`函数中,我们创建了一个二叉树,并调用这两个函数来计算节点总数和深度,并输出结果。
阅读全文
相关推荐
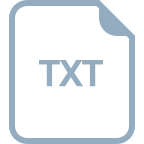
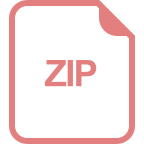















