vue 使用input与a实现搜索
时间: 2023-06-01 09:07:41 浏览: 103
可以使用input标签与a标签的结合实现搜索功能。在input标签中输入搜索关键字,然后点击a标签的链接跳转到搜索结果页面。这个方法通常被称为“GET”搜索。除此之外,还可以使用“POST”搜索方式,这需要使用表单和后端处理脚本来实现。
相关问题
vue 使用input与a实现搜索 代码示例
好的,下面是一个简单的 Vue 搜索栏示例,使用了一个 input 和一个 a 标签:
```
<template>
<div>
<input type="text" v-model="searchText">
<a v-bind:href="searchUrl">搜索</a>
</div>
</template>
<script>
export default {
data() {
return {
searchText: '',
searchUrl: 'https://www.baidu.com/s?wd='
}
},
computed: {
encodedSearchText() {
return encodeURIComponent(this.searchText.trim());
}
},
watch: {
encodedSearchText(val) {
this.searchUrl = 'https://www.baidu.com/s?wd=' + val;
}
}
}
</script>
```
在这个示例中,我们将输入的搜索关键字保存在 `searchText` 变量中,利用 Vue 双向数据绑定机制,实现了搜索关键字的实时更新。同时,利用 computed 属性,在输入关键字的过程中,会自动对关键字进行 URL 编码。在 watch 属性中,更新了 a 标签的 href 属性,实现了跳转到搜索结果页面的功能。
ant design vue 怎么使用input搜索table中的数据,并且让搜索关键词高亮,table组件的数据是数组形式,从接口获取,部分字段需要前端转义为汉字,
你可以使用 ant-design-vue 的 Table 组件的筛选功能来实现搜索功能。具体步骤如下:
1. 在你的 Table 组件中加入 `filter-method` 属性,并设置为一个函数,该函数接收两个参数:`value`(搜索关键字)和 `record`(当前行的数据),返回一个布尔值表示该行是否需要显示。
例如:
```html
<a-table :columns="columns" :data-source="dataSource" :filter-method="filterTable"></a-table>
```
```javascript
methods: {
filterTable(value, record) {
// 判断该行数据是否包含搜索关键字
const keys = Object.keys(record)
for (let i = 0; i < keys.length; i++) {
const key = keys[i]
const fieldValue = record[key]
if (String(fieldValue).indexOf(value) !== -1) {
return true
}
}
return false
}
}
```
2. 在搜索关键字输入框中加入 `v-model`,绑定到一个变量上,例如 `searchKeyword`。
```html
<a-input v-model="searchKeyword"></a-input>
```
3. 在你的 Table 组件中加入 `custom-row` 属性,并设置为一个函数,该函数接收一个参数 `record`(当前行的数据),返回一个对象,表示对应行中需要高亮显示的字段以及高亮前缀和后缀。
例如:
```html
<a-table :columns="columns" :data-source="dataSource" :filter-method="filterTable" :custom-row="renderTableRow"></a-table>
```
```javascript
methods: {
renderTableRow(record) {
const keys = Object.keys(record)
const highlightPrefix = '<span style="color: red">'
const highlightSuffix = '</span>'
const highlightedFields = {}
for (let i = 0; i < keys.length; i++) {
const key = keys[i]
const fieldValue = record[key]
const highlightedValue = String(fieldValue).replace(this.searchKeyword, highlightPrefix + this.searchKeyword + highlightSuffix)
highlightedFields[key] = highlightedValue
}
return { children: highlightedFields }
}
}
```
4. 在上面的示例函数中,我们使用了 `String.prototype.replace()` 方法将搜索关键字替换为高亮的 HTML 标签。在你的代码中需要将所有需要高亮的字段都进行类似的处理。同时,还需要在前端将一些字段进行转义为汉字,例如可以使用 Vue 的过滤器。
例如:
```html
<a-table :columns="columns" :data-source="dataSource" :filter-method="filterTable" :custom-row="renderTableRow"></a-table>
```
```javascript
methods: {
renderTableRow(record) {
const keys = Object.keys(record)
const highlightPrefix = '<span style="color: red">'
const highlightSuffix = '</span>'
const highlightedFields = {}
for (let i = 0; i < keys.length; i++) {
const key = keys[i]
const fieldValue = record[key]
let highlightedValue = fieldValue
if (key === 'status') {
highlightedValue = this.$options.filters.statusText(fieldValue)
}
if (key === 'name') {
highlightedValue = this.$options.filters.escapeHtml(fieldValue)
}
highlightedValue = String(highlightedValue).replace(this.searchKeyword, highlightPrefix + this.searchKeyword + highlightSuffix)
highlightedFields[key] = highlightedValue
}
return { children: highlightedFields }
}
},
filters: {
statusText(value) {
if (value === 'pending') {
return '待处理'
} else if (value === 'approved') {
return '已通过'
} else if (value === 'rejected') {
return '已拒绝'
} else {
return '未知'
}
},
escapeHtml(value) {
return value.replace(/&/g, '&').replace(/</g, '<').replace(/>/g, '>')
}
}
```
在上面的示例代码中,我们定义了两个过滤器 `statusText` 和 `escapeHtml`,分别用于将状态值和名称进行转义为汉字和 HTML 实体。在 `renderTableRow` 函数中,我们根据键名来判断需要进行哪些转义操作,并将转义后的值进行高亮处理。最终返回的对象中,我们将高亮后的值作为 `children` 属性返回,这样就能够实现搜索关键字高亮的效果了。
阅读全文
相关推荐
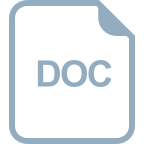
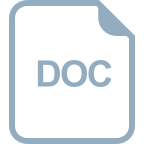
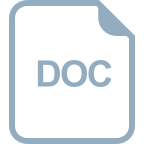
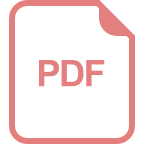
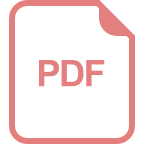
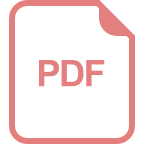
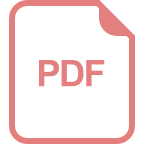
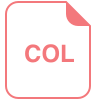








