list(1. for i in range(10))
时间: 2024-05-14 15:15:00 浏览: 30
This code is incorrect and will result in a syntax error.
The correct way to create a list using a for loop is:
```python
my_list = [i for i in range(10)]
```
This creates a list of numbers from 0 to 9.
相关问题
list1=[] sum=0 list1.append(1) list1.append(1) for i in range(2,5): list1[i]=list1[i-2]+list1[i-1] print(list1)
这段代码存在两个问题:
1. 在第一次循环时,list1 的长度为 0,因此无法通过下标访问 list1 中的元素,会导致 IndexError 异常。正确的做法应该是使用 `list1.append(0)` 来扩展列表长度。
2. 在循环中计算斐波那契数列的值时,应该使用 `list1.append(list1[i-2]+list1[i-1])` 来添加新的元素,而不是通过下标修改已有的元素。
修改后的代码如下:
```
list1 = []
sum = 0
list1.append(1)
list1.append(1)
for i in range(2, 5):
list1.append(list1[i-2] + list1[i-1])
print(list1)
```
输出结果为 `[1, 1, 2, 3, 5]`,符合斐波那契数列的定义。
test_loss = 0.0 class_correct = list(0. for i in range(2)) class_total = list(0. for i in range(2)) model.eval() # iterate over test data for data, target in test_loader: # move tensors to GPU if CUDA is available if train_on_gpu: data, target = data.cuda(), target.cuda() # forward pass: compute predicted outputs by passing inputs to the model output = model(data) # calculate the batch loss loss = criterion(output, target) # update test loss test_loss += loss.item()*data.size(0) # convert output probabilities to predicted class _, pred = torch.max(output, 1) # compare predictions to true label correct_tensor = pred.eq(target.data.view_as(pred)) correct = np.squeeze(correct_tensor.numpy()) if not train_on_gpu else np.squeeze(correct_tensor.cpu().numpy()) # calculate test accuracy for each object class for i in range(batch_size): label = target.data[i] class_correct[label] += correct[i].item() class_total[label] += 1 # average test loss test_loss = test_loss/len(test_loader.dataset) print('Test Loss: {:.6f}\n'.format(test_loss))哪里有问题
在代码中没有明显的错误,但有几个注意事项需要考虑:
1. 你需要确保 `test_loader` 是正确加载测试数据的。
2. `model.eval()` 用于将模型设置为评估模式,这会影响到一些层的行为,例如 `BatchNorm` 和 `Dropout`。
3. 确保 `criterion` 是正确的损失函数。
4. 如果你想计算每个类别的准确率,确保你的模型输出维度与类别数目相匹配。
阅读全文
相关推荐
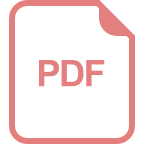













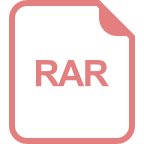
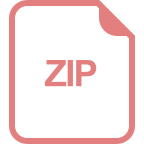