self.sigma1, self.theta1, self.Lambda1, self.psi1, self.gamma1, self.bias1 = self.generate_parameters(channel1//2, in_channels)
时间: 2024-06-07 16:05:46 浏览: 80
这段代码是在生成第一层的参数,其中参数的含义如下:
- `channel1`: 第一层的输出通道数。
- `in_channels`: 输入的通道数。
具体地,这里使用了一个 Gabor 滤波器,其中 `sigma1` 表示高斯函数的标准差,`theta1` 表示 Gabor 滤波器的方向,`Lambda1` 表示 Gabor 滤波器的波长,`psi1` 表示 Gabor 滤波器的相位偏移,`gamma1` 表示 Gabor 滤波器的纵横比,`bias1` 表示 Gabor 滤波器的偏置项。这些参数的具体含义可以参考 Gabor 滤波器的相关资料。
相关问题
import numpy as np from sklearn.datasets import load_iris from sklearn.model_selection import train_test_split import matplotlib.pyplot as plt # 加载 iris 数据 iris = load_iris() # 只选取两个特征和两个类别进行二分类 X = iris.data[(iris.target==0)|(iris.target==1), :2] y = iris.target[(iris.target==0)|(iris.target==1)] # 将标签转化为 0 和 1 y[y==0] = -1 # 将数据集分为训练集和测试集 X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # 实现逻辑回归算法 class LogisticRegression: def __init__(self, lr=0.01, num_iter=100000, fit_intercept=True, verbose=False): self.lr = lr self.num_iter = num_iter self.fit_intercept = fit_intercept self.verbose = verbose def __add_intercept(self, X): intercept = np.ones((X.shape[0], 1)) return np.concatenate((intercept, X), axis=1) def __sigmoid(self, z): return 1 / (1 + np.exp(-z)) def __loss(self, h, y): return (-y * np.log(h) - (1 - y) * np.log(1 - h)).mean() def fit(self, X, y): if self.fit_intercept: X = self.__add_intercept(X) # 初始化参数 self.theta = np.zeros(X.shape[1]) for i in range(self.num_iter): # 计算梯度 z = np.dot(X, self.theta) h = self.__sigmoid(z) gradient = np.dot(X.T, (h - y)) / y.size # 更新参数 self.theta -= self.lr * gradient # 打印损失函数 if self.verbose and i % 10000 == 0: z = np.dot(X, self.theta) h = self.__sigmoid(z) loss = self.__loss(h, y) print(f"Loss: {loss} \t") def predict_prob(self, X): if self.fit_intercept: X = self.__add_intercept(X) return self.__sigmoid(np.dot(X, self.theta)) def predict(self, X, threshold=0.5): return self.predict_prob(X) >= threshold # 训练模型 model = LogisticRegressio
n()
model.fit(X_train, y_train)
# 在测试集上进行预测
y_pred = model.predict(X_test)
# 计算准确率
accuracy = np.sum(y_pred == y_test) / y_test.shape[0]
print(f"Accuracy: {accuracy}")
# 可视化
plt.scatter(X_test[:, 0], X_test[:, 1], c=y_pred)
plt.show()
请问这段代码实现了什么功能?
import numpy as np class Node: j = None theta = None p = None left = None right = None class DecisionTreeBase: def __init__(self, max_depth, feature_sample_rate, get_score): self.max_depth = max_depth self.feature_sample_rate = feature_sample_rate self.get_score = get_score def split_data(self, j, theta, X, idx): idx1, idx2 = list(), list() for i in idx: value = X[i][j] if value <= theta: idx1.append(i) else: idx2.append(i) return idx1, idx2 def get_random_features(self, n): shuffled = np.random.permutation(n) size = int(self.feature_sample_rate * n) selected = shuffled[:size] return selected def find_best_split(self, X, y, idx): m, n = X.shape best_score = float("inf") best_j = -1 best_theta = float("inf") best_idx1, best_idx2 = list(), list() selected_j = self.get_random_features(n) for j in selected_j: thetas = set([x[j] for x in X]) for theta in thetas: idx1, idx2 = self.split_data(j, theta, X, idx) if min(len(idx1), len(idx2)) == 0 : continue score1, score2 = self.get_score(y, idx1), self.get_score(y, idx2) w = 1.0 * len(idx1) / len(idx) score = w * score1 + (1-w) * score2 if score < best_score: best_score = score best_j = j best_theta = theta best_idx1 = idx1 best_idx2 = idx2 return best_j, best_theta, best_idx1, best_idx2, best_score def generate_tree(self, X, y, idx, d): r = Node() r.p = np.average(y[idx], axis=0) if d == 0 or len(idx)<2: return r current_score = self.get_score(y, idx) j, theta, idx1, idx2, score = self.find_best_split(X, y, idx) if score >= current_score: return r r.j = j r.theta = theta r.left = self.generate_tree(X, y, idx1, d-1) r.right = self.generate_tree(X, y, idx2, d-1) return r def fit(self, X, y): self.root = self.generate_tree(X, y, range(len(X)), self.max_depth) def get_prediction(self, r, x): if r.left == None and r.right == None: return r.p value = x[r.j] if value <= r.theta: return self.get_prediction(r.left, x) else: return self.get_prediction(r.right, x) def predict(self, X): y = list() for i in range(len(X)): y.append(self.get_prediction(self.root, X[i])) return np.array(y)
这段代码实现了一个基于决策树的分类器,其中包括以下几个类和方法:
1. Node类:表示决策树节点的类,包括属性j表示节点所选择的特征,属性theta表示节点所选择的特征的阈值,属性p表示节点的预测值,属性left和right分别表示左子树和右子树。
2. DecisionTreeBase类:表示决策树分类器的基类,包括方法__init__()、split_data()、get_random_features()、find_best_split()、generate_tree()、fit()、get_prediction()和predict()。
3. __init__(self, max_depth, feature_sample_rate, get_score)方法:初始化决策树分类器的参数,包括最大深度、特征采样率和评价指标。
4. split_data(self, j, theta, X, idx)方法:根据特征j和阈值theta将数据集X中的数据划分为两部分,返回划分后的两部分数据在数据集X中的索引。
5. get_random_features(self, n)方法:从数据集X中随机选择一定比例的特征,返回特征的索引。
6. find_best_split(self, X, y, idx)方法:在数据集X和标签y中,根据评价指标找到最优的特征和阈值,返回最优特征的索引、最优阈值、划分后的两部分数据在数据集X中的索引以及最优评价指标的值。
7. generate_tree(self, X, y, idx, d)方法:根据数据集X、标签y和索引idx生成一棵决策树,返回根节点。
8. fit(self, X, y)方法:训练决策树分类器,生成决策树。
9. get_prediction(self, r, x)方法:对于输入的数据x,根据决策树节点r的特征和阈值进行判断,选择左子树或右子树,并递归调用get_prediction()方法,直到到达叶子节点返回预测值。
10. predict(self, X)方法:对于输入的数据集X,返回预测值。
阅读全文
相关推荐
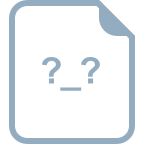
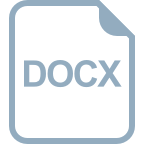
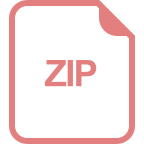







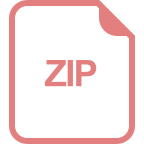
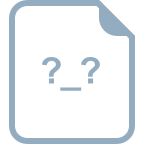
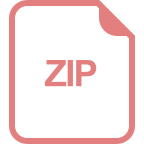
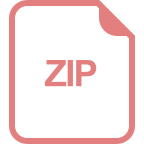
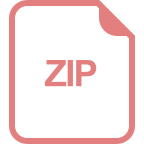