建立一链表结构,每个结点包括职工号, 姓名,部门名和工资(整数)。采用带头结点尾插法建立链表,职工号为0输入结束。然后输入一部门名,删除链表上所有是该部门职工的结点, 输出结果到屏幕上。(建议建链表, 删除信息和输出结果分别用三个自定义函数实现)
时间: 2024-05-18 20:16:38 浏览: 82
好的,我理解了你的问题。下面是一个基于C语言的代码实现。请注意,这是一个简单的示例代码,可能不符合实际应用场景中的需求。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义链表结点结构体
struct employee {
int id; // 职工号
char name[20]; // 姓名
char department[20]; // 部门名
int salary; // 工资
struct employee *next; // 指向下一个结点的指针
};
// 带头结点尾插法建立链表
struct employee *create_list() {
struct employee *head, *p, *q;
head = (struct employee *)malloc(sizeof(struct employee));
head->next = NULL;
q = head;
printf("请依次输入职工号、姓名、部门名和工资,职工号为0输入结束:\n");
do {
p = (struct employee *)malloc(sizeof(struct employee));
scanf("%d", &p->id);
if (p->id == 0) {
free(p);
break;
}
scanf("%s%s%d", p->name, p->department, &p->salary);
q->next = p;
q = p;
} while (1);
q->next = NULL;
return head;
}
// 删除链表上指定部门的职工结点
struct employee *delete_department(struct employee *head, char *department) {
struct employee *p, *q;
p = head->next;
q = head;
while (p != NULL) {
if (strcmp(p->department, department) == 0) {
q->next = p->next;
free(p);
p = q->next;
} else {
q = p;
p = p->next;
}
}
return head;
}
// 输出链表中的所有职工信息
void print_list(struct employee *head) {
struct employee *p;
p = head->next;
printf("职工号\t姓名\t部门名\t工资\n");
while (p != NULL) {
printf("%d\t%s\t%s\t%d\n", p->id, p->name, p->department, p->salary);
p = p->next;
}
}
int main() {
struct employee *head;
char department[20];
head = create_list();
printf("请输入要删除的部门名:\n");
scanf("%s", department);
head = delete_department(head, department);
print_list(head);
return 0;
}
```
这个程序中,我们首先定义了链表结点的数据结构,包括职工号、姓名、部门名和工资等信息。然后,通过带头结点尾插法建立了一个链表。接着,我们实现了一个函数,该函数可以删除链表上指定部门的所有职工结点。最后,我们调用了这两个函数,输出了最终的链表信息。
需要注意的是,这个程序中并没有对输入数据进行严格的错误处理,例如输入的职工号、部门名、工资等信息是否合法没有进行检查。在实际应用中,我们需要根据具体需求进行相应的改进。
阅读全文
相关推荐
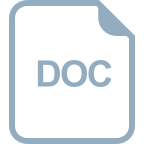
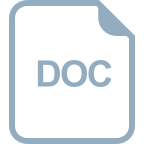
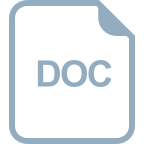















