帮我写一个java红黑树用代码实现
时间: 2023-04-06 07:03:39 浏览: 92
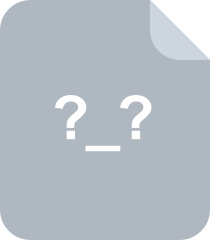
红黑树实现(java代码)
好的,以下是一个简单的Java红黑树实现:
```
public class RedBlackTree<T extends Comparable<T>> {
private static final boolean RED = true;
private static final boolean BLACK = false;
private Node<T> root;
private static class Node<T> {
T key;
Node<T> left, right;
boolean color;
Node(T key, boolean color) {
this.key = key;
this.color = color;
}
}
public void insert(T key) {
root = insert(root, key);
root.color = BLACK;
}
private Node<T> insert(Node<T> h, T key) {
if (h == null) return new Node<>(key, RED);
int cmp = key.compareTo(h.key);
if (cmp < 0) h.left = insert(h.left, key);
else if (cmp > 0) h.right = insert(h.right, key);
else ; // do nothing
if (isRed(h.right) && !isRed(h.left)) h = rotateLeft(h);
if (isRed(h.left) && isRed(h.left.left)) h = rotateRight(h);
if (isRed(h.left) && isRed(h.right)) flipColors(h);
return h;
}
private boolean isRed(Node<T> x) {
if (x == null) return false;
return x.color == RED;
}
private Node<T> rotateLeft(Node<T> h) {
Node<T> x = h.right;
h.right = x.left;
x.left = h;
x.color = h.color;
h.color = RED;
return x;
}
private Node<T> rotateRight(Node<T> h) {
Node<T> x = h.left;
h.left = x.right;
x.right = h;
x.color = h.color;
h.color = RED;
return x;
}
private void flipColors(Node<T> h) {
h.color = RED;
h.left.color = BLACK;
h.right.color = BLACK;
}
}
```
希望这个代码能够帮到你!
阅读全文
相关推荐
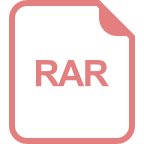
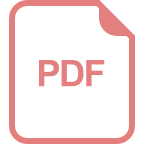
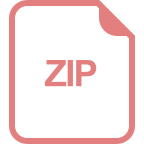
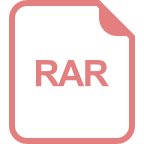
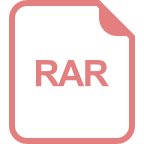
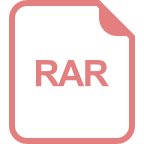
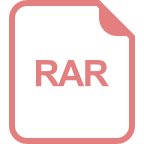
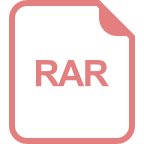
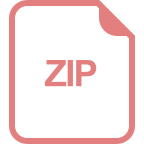
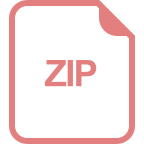
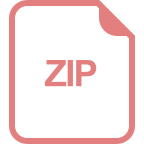
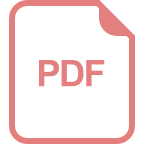
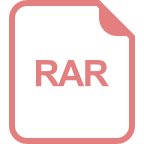