input = torch.ones(size=(batch_size, context_size)).long().to(device)
时间: 2024-05-30 09:09:35 浏览: 79
这行代码创建了一个大小为 `(batch_size, context_size)` 的张量,张量的元素均为1,数据类型为`long`。`.to(device)`将张量移动到指定设备上,其中`device`是一个指定的设备对象,例如`device = torch.device("cuda:0")`表示使用第一个cuda设备。
相关问题
class LSTM(nn.Module): def __init__(self, input_size, hidden_size, num_layers, batch_size, device="cpu"): super().__init__() self.device = device self.input_size = input_size self.hidden_size = hidden_size self.num_layers = num_layers self.batch_size = batch_size self.lstm = nn.LSTM(self.input_size, self.hidden_size, self.num_layers, batch_first=True, bidirectional=False) def forward(self, input_seq): batch_size, seq_len = input_seq.shape[0], input_seq.shape[1] h_0 = torch.randn(self.num_layers, batch_size, self.hidden_size).to(self.device) c_0 = torch.randn(self.num_layers, batch_size, self.hidden_size).to(self.device) output, (h, c) = self.lstm(input_seq, (h_0, c_0)) return output, h
这段代码定义了一个名为 `LSTM` 的类,继承了 `nn.Module` 类,并实现了 `forward()` 方法。在 `forward()` 方法中,输入数据 `input_seq` 被传入 LSTM 模型中进行处理。该方法返回两个值:
- `output`:LSTM 模型在处理输入数据后的输出,包括每个时间步的输出。
- `h`:LSTM 模型最后一个时间步的隐状态,作为后续模型处理的初始状态。
在 `__init__()` 方法中,类的实例变量被初始化,包括:
- `device`:该变量指定了设备类型,默认为 "cpu"。
- `input_size`:该变量指定了输入数据的特征维度。
- `hidden_size`:该变量指定了 LSTM 模型中隐藏层的维度。
- `num_layers`:该变量指定了 LSTM 模型的层数。
- `batch_size`:该变量指定了输入数据的批次大小。
然后,通过 `nn.LSTM` 类创建了一个名为 `lstm` 的 LSTM 模型,该模型的输入特征维度为 `input_size`,隐藏层维度为 `hidden_size`,层数为 `num_layers`,并且设置了 `batch_first=True` 表示输入数据的第一维为批次大小,`bidirectional=False` 表示不使用双向 LSTM。
这个 `LSTM` 类的实例可以用于处理序列数据,并通过前向传播方法 `forward()` 对数据进行处理,返回处理后的输出 `output` 和最后一个时间步的隐状态 `h`。
class my_BiGRU(torch.nn.Module): def __init__(self, input_size, hidden_size, num_layers, output_size, gru_dropout): super(my_BiGRU, self).__init__() self.input_size = input_size self.hidden_size = hidden_size self.num_layers = num_layers self.output_size = output_size self.gru_dropout = gru_dropout self.gru = torch.nn.GRU(input_size, hidden_size, num_layers=num_layers, batch_first=True, bidirectional=True) self.fc = torch.nn.Linear(hidden_size * 2, output_size) def forward(self, x): h0 = torch.zeros(self.num_layers * 2, x.size(0), self.hidden_size).to(x.device) out, _ = self.gru(x, h0) out = self.fc(out[:, -1, :]) return out
这是一个使用双向GRU和全连接层的神经网络模型。该模型的输入大小为input_size,输出大小为output_size。它有num_layers层双向GRU,每层的隐藏状态大小为hidden_size。在GRU层之后,它使用全连接层将GRU的输出转换为所需的输出大小。该模型还使用了dropout来减少过拟合。在forward函数中,它首先将输入x传递给双向GRU,然后将最后一个时间步的输出传递给全连接层以获取最终输出。
阅读全文
相关推荐
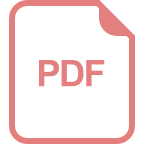










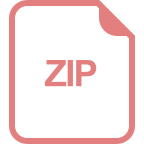

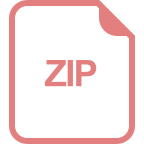

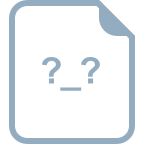