怎么把XGBoost模型转换为C代码
时间: 2024-05-03 10:18:04 浏览: 256
将XGBoost模型转换为C代码可以通过以下步骤实现:
1. 使用XGBoost模型导出函数xgboost::Booster::PredictorToBinary,将模型转换为二进制格式。
2. 使用C++代码读取二进制文件,将模型加载到内存中。
3. 将模型转换为C代码,包括模型参数、树结构和叶节点权重。
4. 将C代码编译成可执行文件或动态链接库,供其他应用程序使用。
下面是一个简单的示例代码,演示如何使用XGBoost C++ API将模型导出为二进制格式并将其加载到内存中:
```c++
#include <xgboost/c_api.h>
#include <iostream>
#include <fstream>
#include <vector>
int main() {
// Load the model from file
std::string model_path = "model.bin";
std::ifstream model_file(model_path, std::ios::binary);
if (!model_file.is_open()) {
std::cerr << "Failed to open model file: " << model_path << std::endl;
return 1;
}
model_file.seekg(0, std::ios::end);
size_t model_size = model_file.tellg();
model_file.seekg(0, std::ios::beg);
std::vector<char> model_data(model_size);
model_file.read(model_data.data(), model_size);
model_file.close();
// Load the binary model into memory
BoosterHandle booster;
XGBoostLoadModelFromMemory(model_data.data(), model_size, &booster);
// Export the binary model to a file
std::string output_path = "model.c";
std::ofstream output_file(output_path, std::ios::binary);
if (!output_file.is_open()) {
std::cerr << "Failed to create output file: " << output_path << std::endl;
return 1;
}
XGBoostDumpModel(booster, 0, 0, "json", output_file);
output_file.close();
// Release the model handle
XGBoostBoosterFree(booster);
return 0;
}
```
此代码将从文件中加载XGBoost模型,将其导出为JSON格式,并将其保存到文件中。您可以使用XGBoost提供的其他导出格式,例如文本格式或二进制格式。如果您需要将模型转换为C代码,则需要解析导出的模型文件并将其转换为C代码。
阅读全文
相关推荐
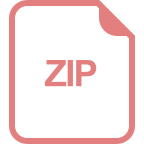
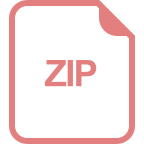
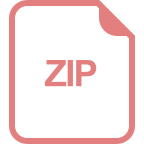





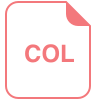
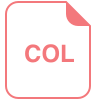

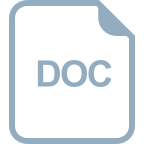
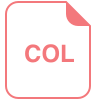
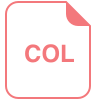
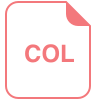
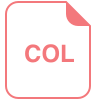
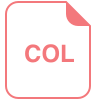
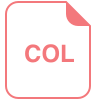
