基于Tensorflow生成Mobielnetv3的代码
时间: 2024-02-25 11:57:18 浏览: 25
以下是一个基于Tensorflow生成Mobilenetv3的代码示例:
```python
import tensorflow as tf
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, Conv2D, DepthwiseConv2D, GlobalAveragePooling2D, Reshape, Dropout, Activation, BatchNormalization, add, Dense
def relu6(x):
return tf.keras.backend.relu(x, max_value=6.0)
def hard_swish(x):
return x * tf.keras.backend.relu(x + 3.0, max_value=6.0) / 6.0
def conv_block(inputs, filters, kernel, strides):
channel_axis = 1 if tf.keras.backend.image_data_format() == 'channels_first' else -1
x = Conv2D(filters, kernel, padding='same', strides=strides)(inputs)
x = BatchNormalization(axis=channel_axis)(x)
return Activation(relu6)(x)
def bottleneck(inputs, filters, kernel, t, s, r=False):
channel_axis = 1 if tf.keras.backend.image_data_format() == 'channels_first' else -1
tchannel = tf.keras.backend.int_shape(inputs)[channel_axis] * t
x = conv_block(inputs, tchannel, (1, 1), (1, 1))
x = DepthwiseConv2D(kernel, strides=(s, s), depth_multiplier=1, padding='same')(x)
x = BatchNormalization(axis=channel_axis)(x)
x = Activation(relu6)(x)
x = Conv2D(filters, (1, 1), strides=(1, 1), padding='same')(x)
x = BatchNormalization(axis=channel_axis)(x)
if r:
x = add([x, inputs])
return x
def MobileNetV3_large(shape=(224, 224, 3), classes=1000):
inputs = Input(shape)
x = conv_block(inputs, 16, (3, 3), strides=(2, 2))
x = bottleneck(x, 16, (3, 3), t=1, s=1, r=True)
x = bottleneck(x, 24, (3, 3), t=4, s=2)
x = bottleneck(x, 24, (3, 3), t=3, s=1, r=True)
x = bottleneck(x, 40, (5, 5), t=3, s=2)
x = bottleneck(x, 40, (5, 5), t=3, s=1, r=True)
x = bottleneck(x, 40, (5, 5), t=3, s=1, r=True)
x = bottleneck(x, 80, (3, 3), t=6, s=2)
x = bottleneck(x, 80, (3, 3), t=2.5, s=1, r=True)
x = bottleneck(x, 80, (3, 3), t=2.3, s=1, r=True)
x = bottleneck(x, 80, (3, 3), t=2.3, s=1, r=True)
x = bottleneck(x, 112, (5, 5), t=6, s=1, r=True)
x = bottleneck(x, 112, (5, 5), t=6, s=1, r=True)
x = bottleneck(x, 160, (5, 5), t=6, s=2, r=True)
x = bottleneck(x, 160, (5, 5), t=6, s=1, r=True)
x = bottleneck(x, 160, (5, 5), t=6, s=1, r=True)
x = conv_block(x, 960, (1, 1), strides=(1, 1))
x = GlobalAveragePooling2D()(x)
x = Reshape((1, 1, 960))(x)
x = Dropout(0.2)(x)
x = Conv2D(classes, (1, 1), padding='same')(x)
x = Activation('softmax')(x)
x = Reshape((classes,))(x)
model = Model(inputs, x)
return model
```
这个代码示例定义了一个名为`MobileNetV3_large`的函数,用于创建一个大型的Mobilenetv3模型。在函数中,我们定义了`conv_block`和`bottleneck`两个函数,用于构建模型的基础组件。然后,我们按照Mobilenetv3的结构,使用这些组件构建了一个大型的模型。最后,我们将模型编译并返回。
相关推荐
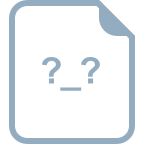
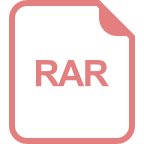















