解释这段代码:int main() { char str[N]; while (fgets(str, N, stdin)) { if (str[0] == '\n') break; str[strlen(str) - 1] = '\0'; Insert(str); } //getchar(); while (fgets(str, N, stdin)) { int len = strlen(str); if (str[len - 1] == '\n') str[strlen(str) - 1] = '\0'; int res = Find(str); if (res > 0) cout << "YES" << endl; else cout << "NO" << endl; } return 0; }
时间: 2024-04-06 08:33:13 浏览: 17
这段代码是一个基于字典树的字符串匹配程序,可以用于查询一些字符串是否存在于一个大字符串集合中。下面是代码的解释:
1. 创建一个字符数组 str,用于存储输入的字符串。
2. 使用 while 循环读入字符串,并调用 Insert 函数将其插入到字典树中。
3. 如果输入的字符串是空行,则跳出循环。
4. 再次使用 while 循环读入字符串,并调用 Find 函数查找该字符串是否在字典树中。
5. 如果查找到了该字符串,则输出 YES,否则输出 NO。
6. 返回 0,表示程序执行成功。
其中,N 是一个预定义的常量,表示字符串的最大长度。Insert 函数是向字典树中插入一个字符串的函数,Find 函数是查找一个字符串是否在字典树中的函数。在这段代码中,使用 fgets 函数读入字符串,可以避免因为输入过长而导致的缓冲区溢出问题。另外,将字符串中的换行符替换为 '\0',可以去掉字符串末尾的换行符,从而方便后续的处理。
相关问题
#include<stdio.h> #include<string.h> int strcompare(const char * str1, const char * str); int main() {char str1[30],str2[30],*p,*q; int a=0; p=str1; q=str2; fgets(str1,30,stdin); fgets(str2,30,stdin); a=strcompare(p, q); printf("%d",a); return 0; } int strcompare(const char * str1, const char * str) {int i; while(*str1==*str&&*str1!='\0'&&*str!='\0') { str1++; str++; } i=*str1-*str; return i; }求ASCLL的差值,检查有什么错误
代码中存在以下问题:
1. fgets()函数会在输入的字符串末尾添加一个换行符,需要在比较之前去除换行符。
2. 当字符串长度相等时,应该返回0,但是该代码返回的是ASCII码的差值,因此需要特判这种情况。
修改后的代码如下:
```
#include<stdio.h>
#include<string.h>
int strcompare(const char * str1, const char * str2);
int main() {
char str1[30],str2[30];
int a=0;
fgets(str1,30,stdin);
fgets(str2,30,stdin);
a=strcompare(str1, str2);
printf("%d",a);
return 0;
}
int strcompare(const char * str1, const char * str2) {
int i;
while(*str1==*str2&&*str1!='\0'&&*str2!='\0') {
str1++;
str2++;
}
if (*str1 == *str2) {
return 0;
}
i=*str1-*str2;
return i;
}
```
注意,这里使用了字符串指针而不是字符指针,因为我们需要比较的是两个字符串,而不是两个字符。
将string类改为char,保持输入的作用不被改变:int main() { string str; map<string, int>m; while (getline(cin,str)) { int len = str.length(); if (!len) break; for(int i = len; i > 0; i--) { string str1 = str.substr(0, i); m[str1]++; } } while (getline(cin, str)) { if (m[str] > 0) cout << "YES" << endl; else cout << "NO" << endl; } return 0; }
可以将上述代码中的string类型改为char类型,并且保持输入的作用不被改变,具体修改如下:
```c++
#include <iostream>
#include <map>
#include <cstring>
using namespace std;
int main() {
char str[1001];
map<string, int> m;
while (fgets(str, 1001, stdin)) {
int len = strlen(str);
if (len == 1 && str[0] == '\n') break;
for (int i = len; i > 0; i--) {
str[i] = '\0';
m[str]++; // 将char类型的字符串转为string类型的字符串
}
}
while (fgets(str, 1001, stdin)) {
int len = strlen(str);
if (len > 0 && str[len - 1] == '\n') str[len - 1] = '\0';
if (m[string(str)] > 0) cout << "YES" << endl; // 将char类型的字符串转为string类型的字符串
else cout << "NO" << endl;
}
return 0;
}
```
在上述修改中,我们将string类型的字符串改为了char类型的字符串,同时保持输入的作用不被改变。在第一个while循环中,我们使用了fgets()函数来获取输入的字符串,fgets()函数可以读取一行字符串,并将其存储到指定的字符数组中。在读取每行字符串后,我们使用for循环逐步截取字符串,并将其插入到map中,这里需要注意的是,我们需要在每个截取的字符串后面添加'\0',以确保字符串的正确性。在第二个while循环中,我们同样使用fgets()函数来获取输入的字符串,并且需要去除字符串末尾的'\n',然后将其转化为string类型的字符串,再到map中查找是否存在,最后输出结果。
相关推荐
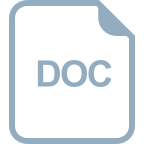
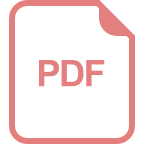
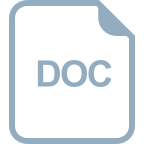













