用一段c语言实现以下功能,输入10个学生5门课的成绩,分别用函数实现下列功能,计算每个学生平均分,找出每门课的平均分,找出所有50个分数中最高的分数所对应的学生和课程
时间: 2024-01-01 11:05:28 浏览: 70
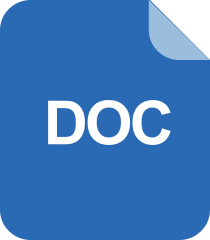
2021年c语言题库.doc
好的,以下是实现该功能的 C 语言代码:
```c
#include <stdio.h>
// 计算每个学生平均分
void calc_student_avg(float score[][5], float student_avg[]) {
for (int i = 0; i < 10; i++) {
float sum = 0.0;
for (int j = 0; j < 5; j++) {
sum += score[i][j];
}
student_avg[i] = sum / 5.0;
}
}
// 找出每门课的平均分
void calc_course_avg(float score[][5], float course_avg[]) {
for (int j = 0; j < 5; j++) {
float sum = 0.0;
for (int i = 0; i < 10; i++) {
sum += score[i][j];
}
course_avg[j] = sum / 10.0;
}
}
// 找出所有50个分数中最高的分数所对应的学生和课程
void find_highest_score(float score[][5], int *student, int *course) {
float highest_score = -1.0;
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 5; j++) {
if (score[i][j] > highest_score) {
highest_score = score[i][j];
*student = i;
*course = j;
}
}
}
}
int main() {
float score[10][5];
float student_avg[10];
float course_avg[5];
// 输入学生成绩
for (int i = 0; i < 10; i++) {
printf("请输入第 %d 个学生的五门课成绩:\n", i+1);
for (int j = 0; j < 5; j++) {
scanf("%f", &score[i][j]);
}
}
// 计算每个学生平均分
calc_student_avg(score, student_avg);
printf("\n每个学生的平均分为:\n");
for (int i = 0; i < 10; i++) {
printf("第 %d 个学生的平均分为:%.2f\n", i+1, student_avg[i]);
}
// 计算每门课的平均分
calc_course_avg(score, course_avg);
printf("\n每门课的平均分为:\n");
for (int j = 0; j < 5; j++) {
printf("第 %d 门课的平均分为:%.2f\n", j+1, course_avg[j]);
}
// 找出所有50个分数中最高的分数所对应的学生和课程
int student, course;
find_highest_score(score, &student, &course);
printf("\n最高分数为:%.2f,对应的学生为第 %d 个,课程为第 %d 门。\n", score[student][course], student+1, course+1);
return 0;
}
```
以上代码会按照以下步骤运行:
1. 提示用户依次输入每个学生的五门课成绩,将其保存在二维数组 `score` 中;
2. 调用 `calc_student_avg` 函数计算每个学生的平均分,将结果保存在一维数组 `student_avg` 中,并打印每个学生的平均分;
3. 调用 `calc_course_avg` 函数计算每门课的平均分,将结果保存在一维数组 `course_avg` 中,并打印每门课的平均分;
4. 调用 `find_highest_score` 函数找出所有50个分数中最高的分数所对应的学生和课程,打印最高分数以及对应的学生和课程。
注意:以上代码假设每个学生都有五门课,如果实际情况不同,需要相应地修改代码。
阅读全文
相关推荐
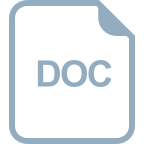
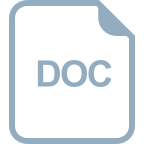
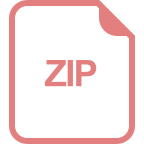





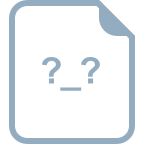
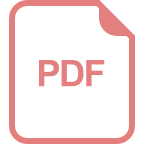
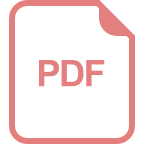
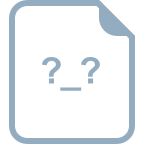
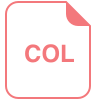





