网络选拔赛题库系统java实例
时间: 2023-07-05 16:27:09 浏览: 161
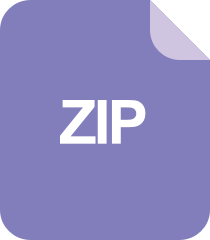
是基于java开发的在线题库系统
以下是一个基本的网络选拔赛题库系统的Java示例:
```
import java.util.ArrayList;
import java.util.Scanner;
public class ContestSystem {
// A list of ContestQuestion objects to store the questions in the contest
private static ArrayList<ContestQuestion> contestQuestions = new ArrayList<>();
// A Scanner object to read input from the user
private static Scanner scanner = new Scanner(System.in);
public static void main(String[] args) {
// Display a welcome message
System.out.println("Welcome to the Contest Question System!");
// Prompt the user to enter the number of questions in the contest
System.out.print("Please enter the number of questions in the contest: ");
int numQuestions = scanner.nextInt();
// Read in each question and add it to the contestQuestions list
for (int i = 1; i <= numQuestions; i++) {
System.out.println("Question " + i + ":");
System.out.print("Enter the question text: ");
String questionText = scanner.next();
System.out.print("Enter the answer: ");
String answer = scanner.next();
ContestQuestion question = new ContestQuestion(questionText, answer);
contestQuestions.add(question);
}
// Display the menu of options
System.out.println();
System.out.println("Please select an option from the menu below:");
System.out.println("1. Take the contest");
System.out.println("2. View the questions in the contest");
System.out.println("3. Exit");
// Read the user's choice from the keyboard
int choice = scanner.nextInt();
// Process the user's choice
switch (choice) {
case 1:
takeContest();
break;
case 2:
viewQuestions();
break;
case 3:
System.out.println("Goodbye!");
break;
default:
System.out.println("Invalid choice. Please try again.");
break;
}
// Close the Scanner object
scanner.close();
}
public static void takeContest() {
// Initialize the user's score to 0
int score = 0;
// Iterate over each question in the contestQuestions list
for (int i = 0; i < contestQuestions.size(); i++) {
ContestQuestion question = contestQuestions.get(i);
System.out.println("Question " + (i+1) + ": " + question.getQuestionText());
System.out.print("Your answer: ");
String userAnswer = scanner.next();
if (userAnswer.equals(question.getAnswer())) {
System.out.println("Correct!");
score++;
} else {
System.out.println("Incorrect. The correct answer was: " + question.getAnswer());
}
}
// Display the user's final score
System.out.println("Your final score is: " + score + "/" + contestQuestions.size());
}
public static void viewQuestions() {
// Iterate over each question in the contestQuestions list and display the question text
for (int i = 0; i < contestQuestions.size(); i++) {
ContestQuestion question = contestQuestions.get(i);
System.out.println("Question " + (i+1) + ": " + question.getQuestionText());
}
}
}
class ContestQuestion {
private String questionText;
private String answer;
public ContestQuestion(String questionText, String answer) {
this.questionText = questionText;
this.answer = answer;
}
public String getQuestionText() {
return questionText;
}
public String getAnswer() {
return answer;
}
}
```
该示例实现了一个简单的网络选拔赛题库系统,用户可以添加他们想要的问题和答案,并选择参加比赛或查看问题列表。在参加比赛时,系统会迭代每个问题,提示用户输入答案,然后根据答案是否正确更新用户的得分。在查看问题列表时,系统会显示每个问题的文本。请注意,这里省略了一些边缘情况(例如,用户输入的不正确的选择或答案),这是为了简化示例。
阅读全文
相关推荐
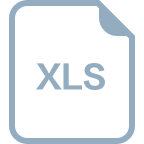
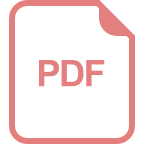
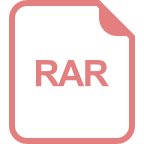
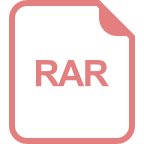
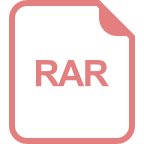
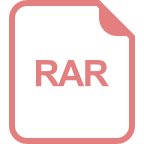
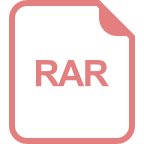
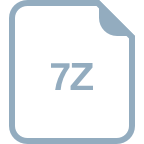
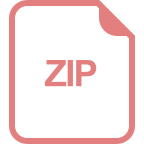
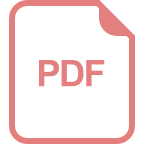
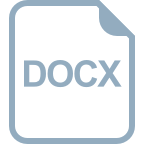
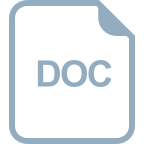
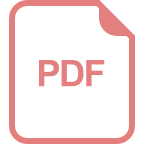