用C++编制用Lagrange插值多项式和多项式拟合最小二乘法的方法计算经过三个数据点(1,2)、(-1,1)、(2,3)的抛物线和拟合三个数据点(1,2)、(-1,1)、(2,3)的抛物线的程序
时间: 2023-10-09 15:13:52 浏览: 82
Lagrange插值多项式的程序:
```c
#include <stdio.h>
double lagrange(double x[], double y[], int n, double x0)
{
double result = 0.0;
for (int i = 0; i < n; i++) {
double term = y[i];
for (int j = 0; j < n; j++) {
if (j != i) {
term *= (x0 - x[j]) / (x[i] - x[j]);
}
}
result += term;
}
return result;
}
int main()
{
double x[] = {1.0, -1.0, 2.0};
double y[] = {2.0, 1.0, 3.0};
int n = 3;
double x0 = 0.0;
double y0 = lagrange(x, y, n, x0);
printf("The value of y at x = %.2f is %.2f\n", x0, y0);
return 0;
}
```
多项式拟合最小二乘法的程序:
```c
#include <stdio.h>
#include <math.h>
#define MAXN 100
void least_squares(double x[], double y[], int n, double *a, double *b, double *c)
{
double sx = 0.0, sy = 0.0, sxx = 0.0, sxy = 0.0, sxxx = 0.0, sxxy = 0.0, sxxxx = 0.0, sxxyy = 0.0;
for (int i = 0; i < n; i++) {
sx += x[i];
sy += y[i];
sxx += x[i] * x[i];
sxy += x[i] * y[i];
sxxx += x[i] * x[i] * x[i];
sxxy += x[i] * x[i] * y[i];
sxxxx += x[i] * x[i] * x[i] * x[i];
sxxyy += x[i] * x[i] * y[i] * y[i];
}
double A[3][3] = {
{n, sx, sxx},
{sx, sxx, sxxx},
{sxx, sxxx, sxxxx}
};
double B[3] = {sy, sxy, sxxyy};
double detA = A[0][0] * (A[1][1] * A[2][2] - A[1][2] * A[2][1])
- A[0][1] * (A[1][0] * A[2][2] - A[1][2] * A[2][0])
+ A[0][2] * (A[1][0] * A[2][1] - A[1][1] * A[2][0]);
double invA[3][3] = {
{(A[1][1] * A[2][2] - A[1][2] * A[2][1]) / detA, (A[0][2] * A[2][1] - A[0][1] * A[2][2]) / detA, (A[0][1] * A[1][2] - A[0][2] * A[1][1]) / detA},
{(A[1][2] * A[2][0] - A[1][0] * A[2][2]) / detA, (A[0][0] * A[2][2] - A[0][2] * A[2][0]) / detA, (A[0][2] * A[1][0] - A[0][0] * A[1][2]) / detA},
{(A[1][0] * A[2][1] - A[1][1] * A[2][0]) / detA, (A[0][1] * A[2][0] - A[0][0] * A[2][1]) / detA, (A[0][0] * A[1][1] - A[0][1] * A[1][0]) / detA}
};
double C[3] = {0.0};
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
C[i] += invA[i][j] * B[j];
}
}
*a = C[0];
*b = C[1];
*c = C[2];
}
int main()
{
double x[] = {1.0, -1.0, 2.0};
double y[] = {2.0, 1.0, 3.0};
int n = 3;
double a, b, c;
least_squares(x, y, n, &a, &b, &c);
printf("The fitting curve is y = %.2f x^2 + %.2f x + %.2f\n", a, b, c);
return 0;
}
```
阅读全文
相关推荐
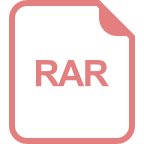



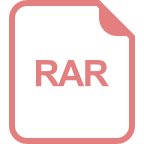
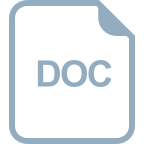
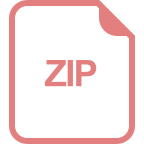
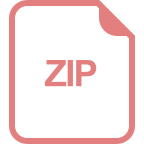
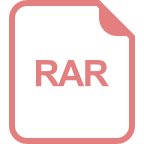
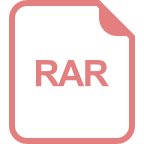
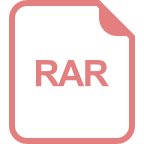
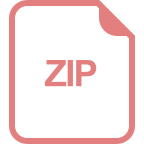
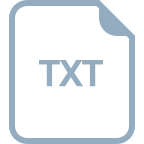
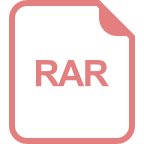
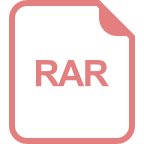
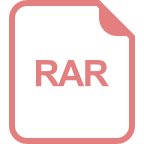
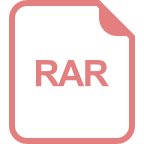