c++完成Lagrange插值多项式以及使用graphics.h库绘图
时间: 2023-08-17 20:05:05 浏览: 93
Lagrange插值多项式是一种数值分析方法,用于计算通过一系列已知数据点的插值函数。插值函数是一个多项式函数,它通过这些数据点,尽可能地拟合这些点。
下面是一个使用C++实现Lagrange插值多项式的示例代码:
```cpp
#include <iostream>
#include <graphics.h>
using namespace std;
// 计算Lagrange插值多项式
double lagrange(double x[], double y[], int n, double xi) {
double result = 0.0;
for (int i = 0; i < n; i++) {
double term = y[i];
for (int j = 0; j < n; j++) {
if (j != i) {
term *= (xi - x[j]) / (x[i] - x[j]);
}
}
result += term;
}
return result;
}
int main() {
int gd = DETECT, gm;
initgraph(&gd, &gm, "");
int n;
cout << "Enter the number of data points: ";
cin >> n;
double x[n], y[n];
for (int i = 0; i < n; i++) {
cout << "Enter the value of x" << i << ": ";
cin >> x[i];
cout << "Enter the value of y" << i << ": ";
cin >> y[i];
}
// 绘制原始数据点
setcolor(RED);
for (int i = 0; i < n; i++) {
circle(x[i], y[i], 5);
}
// 绘制插值多项式
setcolor(BLUE);
for (double i = x[0]; i <= x[n - 1]; i += 0.01) {
double yi = lagrange(x, y, n, i);
putpixel(i, yi, BLUE);
}
getch();
closegraph();
return 0;
}
```
在上面的代码中,我们使用了graphics.h库来绘制插值多项式及原始数据点。运行程序后,它会提示您输入要使用的数据点数。然后,您需要输入每个数据点的x和y值。程序将绘制原始数据点和插值多项式,并在屏幕上显示结果。
注意:graphics.h库在一些编译器中已经不再支持,请根据您的编译器文档了解如何使用。
相关推荐
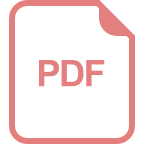
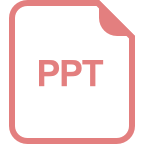
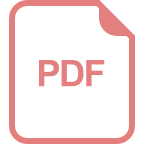














