c++完成Lagrange插值多项式以及使用绘图
时间: 2023-08-17 17:05:05 浏览: 145
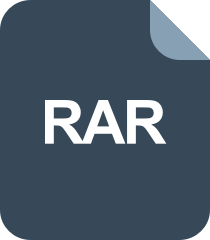
Lagrange插值多项式c语言版

Lagrange插值多项式是一种常用于数据拟合的方法,可以通过已知的数据点来构造一个多项式函数,从而对未知数据进行预测。以下是C++实现Lagrange插值多项式的代码:
```c++
#include <iostream>
#include <vector>
#include <cmath>
#include <fstream>
#include "gnuplot_i.hpp" //使用Gnuplot进行绘图
using namespace std;
//定义数据点结构体
struct point {
double x; //自变量
double y; //因变量
};
//Lagrange插值多项式函数
double Lagrange(vector<point> &data, double x) {
double result = 0;
for (int i = 0; i < data.size(); i++) {
double term = data[i].y;
for (int j = 0; j < data.size(); j++) {
if (j != i) {
term *= (x - data[j].x) / (data[i].x - data[j].x);
}
}
result += term;
}
return result;
}
int main() {
//定义数据点
vector<point> data{{0, 1}, {1, 0}, {2, -1}, {3, 0}, {4, 1}};
//绘制原始数据点
Gnuplot gp;
gp << "set title 'Lagrange Interpolation Polynomial'\n";
gp << "set key off\n";
gp << "plot '-' with points pointtype 6 title 'Data Points'\n";
for (int i = 0; i < data.size(); i++) {
gp << data[i].x << " " << data[i].y << "\n";
}
gp << "e\n";
//计算并绘制插值多项式
double xmin = data[0].x;
double xmax = data[data.size() - 1].x;
double step = 0.01;
gp << "set xrange [" << xmin << ":" << xmax << "]\n";
gp << "plot '-' with lines title 'Lagrange Interpolation Polynomial'\n";
for (double x = xmin; x <= xmax; x += step) {
gp << x << " " << Lagrange(data, x) << "\n";
}
gp << "e\n";
return 0;
}
```
代码中使用了Gnuplot库进行绘图,需要提前安装和配置。运行程序后,将会绘制出原始数据点和插值多项式的图像。
阅读全文
相关推荐
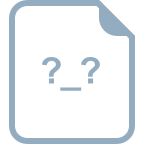
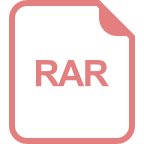












