springboot整合mq
时间: 2023-09-04 16:12:51 浏览: 48
Spring Boot整合MQ(消息队列)可以使用Spring Boot集成的JMS(Java消息服务)或AMQP(高级消息队列协议)实现。下面是使用AMQP的步骤:
1. 添加AMQP依赖
在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
```
2. 配置连接信息
在application.properties文件中添加以下配置:
```properties
spring.rabbitmq.host=localhost
spring.rabbitmq.port=5672
spring.rabbitmq.username=guest
spring.rabbitmq.password=guest
```
3. 创建消息发送者
```java
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class MessageSender {
@Autowired
private RabbitTemplate rabbitTemplate;
public void sendMessage(String message) {
rabbitTemplate.convertAndSend("my-exchange", "my-routing-key", message);
}
}
```
4. 创建消息接收者
```java
import org.springframework.amqp.rabbit.annotation.RabbitListener;
import org.springframework.stereotype.Component;
@Component
public class MessageReceiver {
@RabbitListener(queues = "my-queue")
public void receiveMessage(String message) {
System.out.println("Received message: " + message);
}
}
```
5. 配置消息队列
```java
import org.springframework.amqp.core.Binding;
import org.springframework.amqp.core.BindingBuilder;
import org.springframework.amqp.core.Queue;
import org.springframework.amqp.core.TopicExchange;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class RabbitMQConfig {
@Bean
public Queue myQueue() {
return new Queue("my-queue");
}
@Bean
public TopicExchange myExchange() {
return new TopicExchange("my-exchange");
}
@Bean
public Binding binding(Queue myQueue, TopicExchange myExchange) {
return BindingBuilder.bind(myQueue).to(myExchange).with("my-routing-key");
}
}
```
6. 测试
创建一个Spring Boot应用程序,然后使用MessageSender发送消息,MessageReceiver将接收到该消息。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application implements CommandLineRunner {
@Autowired
private MessageSender messageSender;
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Override
public void run(String... args) throws Exception {
messageSender.sendMessage("Hello, world!");
}
}
```
可以在控制台看到以下输出:
```
Received message: Hello, world!
```
相关推荐
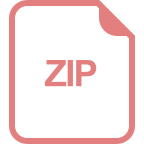














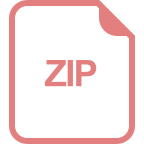