设银行有四个服务窗口,一个等待队列,每个窗口均可以办理存款、取款、挂失、还贷业务,每种业务所需的服务时间不同,优先级不同。客户到达银行后,先到打号机上打号,号票上包括到达时间、编号和需要办理的业务,然后在银行内等候。当任一服务窗口空闲时,处理等候客户中优先级最高,排在最前面的客户的业务。写一个上述银行业务的模拟系统,通过模拟方法求出客户在银行内逗留的平均时间和每个窗口办理的客户数及办理的每种业务数。 基本要求:每个客户到达银行的时间和需要办理的业务随机产生,输出一天客户在银行的平均逗留时间和每个窗口每天办理的客户数和每种业务数。
时间: 2023-11-28 19:49:24 浏览: 53
这是一个典型的排队论问题,可以通过模拟来解决。我们可以使用 Python 编写一个程序来模拟银行的业务流程。
首先,我们需要定义一些参数和变量,如服务窗口数量、等待队列、客户到达时间间隔、客户办理业务需要的时间、客户等待队列中的优先级等级、银行营业时间等。
```python
import random
# 定义参数和变量
NUM_WINDOWS = 4 # 服务窗口数量
WAITING_QUEUE = [] # 等待队列
ARRIVAL_INTERVAL = [1, 3] # 客户到达时间间隔
SERVING_TIME = [[1, 5], [2, 10], [3, 15], [5, 20]] # 客户办理业务需要的时间
PRIORITY_LEVEL = [1, 2, 3, 4] # 客户等待队列中的优先级等级
BANK_HOURS = 8 * 60 # 银行营业时间(分钟)
```
然后,我们需要定义一些函数来模拟客户的到达、等待和离开过程,以及窗口的服务过程。
```python
# 客户到达
def customer_arrival(current_time):
global WAITING_QUEUE
arrival_time = current_time + random.randint(ARRIVAL_INTERVAL[0], ARRIVAL_INTERVAL[1])
service_type = random.randint(0, len(SERVING_TIME) - 1)
priority = random.choice(PRIORITY_LEVEL)
customer = {
"arrival_time": arrival_time,
"service_type": service_type,
"priority": priority
}
WAITING_QUEUE.append(customer)
# 客户等待
def customer_waiting(current_time):
global WAITING_QUEUE
if len(WAITING_QUEUE) > 0:
highest_priority = max(customer["priority"] for customer in WAITING_QUEUE)
waiting_customers = [customer for customer in WAITING_QUEUE if customer["priority"] == highest_priority]
serving_customer = min(waiting_customers, key=lambda x: x["arrival_time"])
WAITING_QUEUE.remove(serving_customer)
service_time = SERVING_TIME[serving_customer["service_type"]][random.randint(0, 1)]
return {
"customer": serving_customer,
"service_time": service_time
}
else:
return None
# 窗口服务
def window_serving(current_time):
serving_customers = []
for i in range(NUM_WINDOWS):
if len(WAITING_QUEUE) == 0:
break
if windows[i]["end_time"] <= current_time:
serving_customer = customer_waiting(current_time)
if serving_customer:
windows[i]["end_time"] = current_time + serving_customer["service_time"]
serving_customers.append(serving_customer)
return serving_customers
# 客户离开
def customer_departure(serving_customers):
global total_waiting_time, total_customers, total_services
for customer in serving_customers:
total_waiting_time += customer["customer"]["arrival_time"] - start_time
total_customers += 1
total_services[customer["customer"]["service_type"]] += 1
```
最后,我们可以编写主程序来模拟整个银行业务流程,并输出统计数据。
```python
# 初始化窗口状态
windows = [{"end_time": 0} for i in range(NUM_WINDOWS)]
# 初始化统计数据
start_time = 0
current_time = start_time
total_waiting_time = 0
total_customers = 0
total_services = [0] * len(SERVING_TIME)
# 模拟银行业务流程
while current_time < BANK_HOURS:
customer_arrival(current_time)
serving_customers = window_serving(current_time)
customer_departure(serving_customers)
current_time += 1
# 输出统计数据
average_waiting_time = total_waiting_time / total_customers
print("客户在银行的平均逗留时间:%.2f 分钟" % average_waiting_time)
print("每个窗口每天办理的客户数:%.2f" % (total_customers / NUM_WINDOWS))
for i in range(len(SERVING_TIME)):
print("窗口每种业务数:%d" % (total_services[i] / NUM_WINDOWS))
```
完整代码如下:
相关推荐
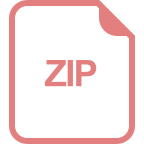





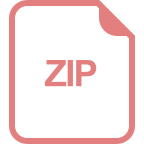
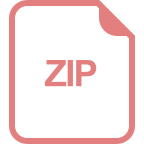
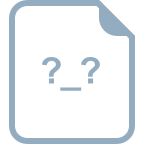
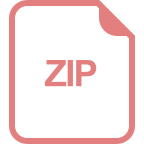
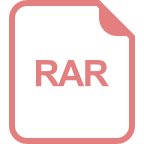
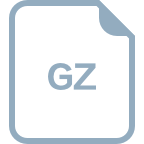