用Python生成densenet例程
时间: 2024-04-10 13:10:54 浏览: 182
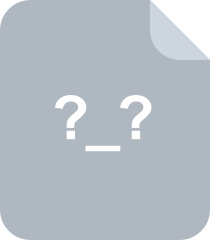
keras_densenet161_finetune.py例子
以下是使用Python生成DenseNet的代码示例:
```python
import tensorflow as tf
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, Dense, Conv2D, Concatenate, BatchNormalization, Activation, GlobalAveragePooling2D
def dense_block(x, num_layers, growth_rate):
for i in range(num_layers):
x1 = BatchNormalization()(x)
x1 = Activation('relu')(x1)
x1 = Conv2D(filters=growth_rate, kernel_size=(3, 3), padding='same')(x1)
x = Concatenate()([x, x1])
return x
def transition_layer(x, compression_factor):
num_filters = int(x.shape[-1] * compression_factor)
x = BatchNormalization()(x)
x = Activation('relu')(x)
x = Conv2D(filters=num_filters, kernel_size=(1, 1), padding='same')(x)
x = tf.keras.layers.AveragePooling2D(pool_size=(2, 2), strides=(2, 2))(x)
return x
def densenet(input_shape=(224, 224, 3), num_classes=1000, growth_rate=32, num_layers_per_block=[6,12,24,16], compression_factor=0.5):
input_layer = Input(shape=input_shape)
x = Conv2D(filters=64, kernel_size=(7, 7), strides=(2, 2), padding='same')(input_layer)
x = BatchNormalization()(x)
x = Activation('relu')(x)
x = tf.keras.layers.MaxPooling2D(pool_size=(3, 3), strides=(2, 2))(x)
for num_layers in num_layers_per_block:
x = dense_block(x, num_layers=num_layers, growth_rate=growth_rate)
x = transition_layer(x, compression_factor=compression_factor)
x = BatchNormalization()(x)
x = Activation('relu')(x)
x = GlobalAveragePooling2D()(x)
output_layer = Dense(units=num_classes, activation='softmax')(x)
model = Model(inputs=input_layer, outputs=output_layer)
return model
model = densenet()
model.summary()
```
在此示例中,我们定义了一个名为`densenet`的函数,该函数接受输入形状、类别数、增长率、每个块中的层数和压缩因子等参数。在函数内部,我们首先定义了输入层,然后使用一些卷积、归一化和激活层对输入进行预处理。接下来,我们使用`dense_block`函数和`transition_layer`函数来定义整个DenseNet的结构。最后,我们定义了一个全局平均池化和一个全连接层来输出预测。
使用`model.summary()`函数可以查看模型的详细结构。
阅读全文
相关推荐
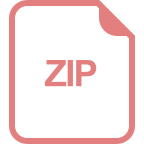
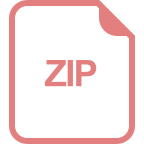
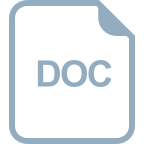
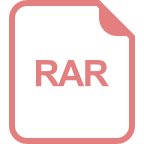
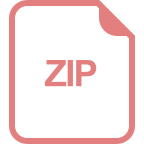
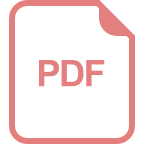
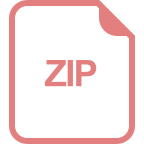
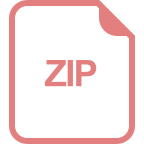
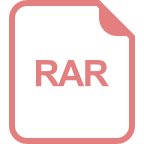
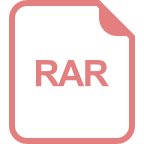
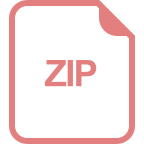
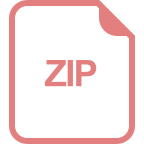
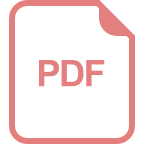
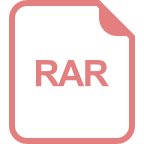
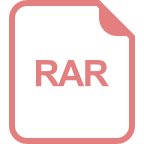
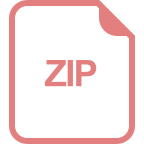