对数据集构建一个分类器,测试分类器性能,列表展示分类器性能指标,画出ROC曲线的python代码
时间: 2024-01-23 17:01:35 浏览: 32
好的,我来给你提供一份Python代码,可以对数据集构建一个分类器,测试分类器性能,列表展示分类器性能指标,画出ROC曲线。
首先,我们需要使用scikit-learn库中的load_iris函数载入数据集,然后将数据集分为训练集和测试集:
```python
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
# 载入数据集
iris = load_iris()
X = iris.data
y = iris.target
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
```
接下来,我们可以使用scikit-learn库中的DecisionTreeClassifier类构建一个决策树分类器,并对其进行训练和测试:
```python
from sklearn.tree import DecisionTreeClassifier
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score, roc_curve, auc
# 构建决策树分类器
clf = DecisionTreeClassifier()
# 训练分类器
clf.fit(X_train, y_train)
# 测试分类器
y_pred = clf.predict(X_test)
```
然后,我们可以使用scikit-learn库中的各种评估指标来评估分类器的性能:
```python
# 计算准确率
accuracy = accuracy_score(y_test, y_pred)
# 计算精确率
precision = precision_score(y_test, y_pred, average='macro')
# 计算召回率
recall = recall_score(y_test, y_pred, average='macro')
# 计算F1-score
f1 = f1_score(y_test, y_pred, average='macro')
# 输出性能指标
print("Accuracy: {:.2f}%".format(accuracy * 100))
print("Precision: {:.2f}%".format(precision * 100))
print("Recall: {:.2f}%".format(recall * 100))
print("F1-score: {:.2f}%".format(f1 * 100))
```
最后,我们可以使用scikit-learn库中的roc_curve和auc函数计算ROC曲线和AUC值,并使用matplotlib库画出ROC曲线图:
```python
import matplotlib.pyplot as plt
# 计算ROC曲线
y_prob = clf.predict_proba(X_test)
fpr, tpr, _ = roc_curve(y_test, y_prob[:, 1])
roc_auc = auc(fpr, tpr)
# 画出ROC曲线图
plt.figure()
plt.plot(fpr, tpr, color='darkorange', lw=2, label='ROC curve (area = {:.2f})'.format(roc_auc))
plt.plot([0, 1], [0, 1], color='navy', lw=2, linestyle='--')
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver operating characteristic')
plt.legend(loc="lower right")
plt.show()
```
以上就是对数据集构建一个分类器,测试分类器性能,列表展示分类器性能指标,画出ROC曲线的Python代码。
相关推荐
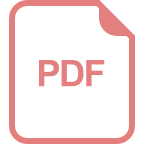
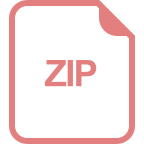
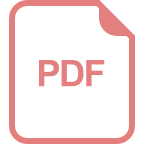














