QGraphicsView绘制同心圆弧
时间: 2023-09-15 11:21:02 浏览: 69
可以通过在QGraphicsView中使用QPainter绘制同心圆弧。
以下是一个示例代码,绘制3个同心圆弧:
```python
from PyQt5.QtWidgets import QGraphicsView, QGraphicsScene
from PyQt5.QtGui import QPainter, QColor, QPen, QBrush
from PyQt5.QtCore import Qt
class MyGraphicsView(QGraphicsView):
def __init__(self, parent=None):
super(MyGraphicsView, self).__init__(parent)
self.scene = QGraphicsScene(self)
self.setScene(self.scene)
def paintEvent(self, event):
painter = QPainter(self.viewport())
# 绘制第一个圆弧
pen = QPen(QColor(255, 0, 0))
pen.setWidth(5)
brush = QBrush(QColor(255, 0, 0, 50))
painter.setPen(pen)
painter.setBrush(brush)
painter.drawArc(50, 50, 200, 200, 0 * 16, 90 * 16)
# 绘制第二个圆弧
pen = QPen(QColor(0, 255, 0))
pen.setWidth(5)
brush = QBrush(QColor(0, 255, 0, 50))
painter.setPen(pen)
painter.setBrush(brush)
painter.drawArc(75, 75, 150, 150, 0 * 16, 90 * 16)
# 绘制第三个圆弧
pen = QPen(QColor(0, 0, 255))
pen.setWidth(5)
brush = QBrush(QColor(0, 0, 255, 50))
painter.setPen(pen)
painter.setBrush(brush)
painter.drawArc(100, 100, 100, 100, 0 * 16, 90 * 16)
if __name__ == '__main__':
import sys
from PyQt5.QtWidgets import QApplication
app = QApplication(sys.argv)
view = MyGraphicsView()
view.show()
sys.exit(app.exec_())
```
在这个示例中,使用QPen和QBrush来设置圆弧的颜色和填充颜色。使用drawArc方法绘制圆弧,其中设置了圆弧的起始角度和角度范围。可以根据需要调整这些参数来绘制不同的圆弧。
相关推荐
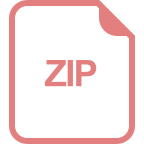
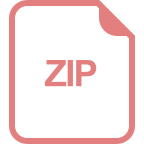














