opencv 识别箭头指向
时间: 2023-07-29 18:06:29 浏览: 208
识别箭头指向的话,可以通过以下步骤实现:
1. 读取图像并将其转换为灰度图像。
2. 使用Canny算子进行边缘检测。
3. 使用霍夫变换(Hough Transform)检测直线。
4. 对于每个检测到的直线,计算它的角度。
5. 根据直线的角度和位置,确定箭头的指向。
6. 绘制箭头指向的方向。
下面是一个大致的OpenCV代码示例,用于实现上述步骤:
```python
import cv2
import numpy as np
# 读取图像并转换为灰度图像
img = cv2.imread('arrow.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 使用Canny算子进行边缘检测
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
# 使用霍夫变换检测直线
lines = cv2.HoughLines(edges, 1, np.pi/180, 200)
# 对于每个检测到的直线,计算它的角度
angles = []
for line in lines:
rho, theta = line[0]
angle = theta * 180 / np.pi
angles.append(angle)
# 根据直线的角度和位置,确定箭头的指向
arrow_direction = None
for angle in angles:
if 45 <= angle <= 135:
arrow_direction = 'up'
elif -45 >= angle >= -135:
arrow_direction = 'down'
elif -45 < angle < 45:
arrow_direction = 'right'
else:
arrow_direction = 'left'
# 绘制箭头指向的方向
cv2.putText(img, arrow_direction, (50, 50), cv2.FONT_HERSHEY_SIMPLEX, 2, (0, 0, 255), 2)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
需要注意的是,上述代码仅供参考,具体的实现方法可能需要根据实际情况进行调整。
相关推荐
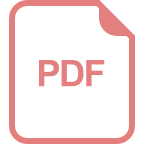
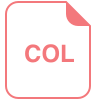
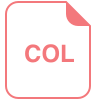
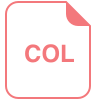
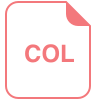
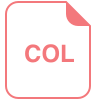









