利用Java GUI相关组件设计实现一个简单的图形用户界面程序代码,并表明程序的名称和主要功能,并写出该程序的UML类图的文本,及该UML类图用visio怎么实现
时间: 2024-03-22 13:39:04 浏览: 15
程序名称:学生信息管理系统
主要功能:该程序用于管理学生信息,包括添加、查询、修改和删除学生信息等功能。
Java GUI程序代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class StudentInfoGUI extends JFrame implements ActionListener {
private JLabel nameLabel, genderLabel, ageLabel, departmentLabel;
private JTextField nameField, ageField;
private JRadioButton maleRadioButton, femaleRadioButton;
private JComboBox<String> departmentComboBox;
private JButton addButton, searchButton, modifyButton, deleteButton;
public StudentInfoGUI() {
// 设置窗口标题
super("学生信息管理系统");
// 初始化组件
nameLabel = new JLabel("姓名:");
genderLabel = new JLabel("性别:");
ageLabel = new JLabel("年龄:");
departmentLabel = new JLabel("院系:");
nameField = new JTextField(20);
ageField = new JTextField(5);
maleRadioButton = new JRadioButton("男");
femaleRadioButton = new JRadioButton("女");
departmentComboBox = new JComboBox<>();
departmentComboBox.addItem("计算机科学与技术");
departmentComboBox.addItem("软件工程");
departmentComboBox.addItem("信息与通信工程");
addButton = new JButton("添加");
searchButton = new JButton("查询");
modifyButton = new JButton("修改");
deleteButton = new JButton("删除");
// 添加组件到容器中
Container container = getContentPane();
container.setLayout(new GridLayout(5, 2));
container.add(nameLabel);
container.add(nameField);
container.add(genderLabel);
container.add(maleRadioButton);
container.add(new JLabel());
container.add(femaleRadioButton);
container.add(ageLabel);
container.add(ageField);
container.add(departmentLabel);
container.add(departmentComboBox);
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new FlowLayout());
buttonPanel.add(addButton);
buttonPanel.add(searchButton);
buttonPanel.add(modifyButton);
buttonPanel.add(deleteButton);
container.add(buttonPanel);
// 注册事件监听器
addButton.addActionListener(this);
searchButton.addActionListener(this);
modifyButton.addActionListener(this);
deleteButton.addActionListener(this);
// 设置窗口大小和可见性
setSize(400, 200);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
// 处理按钮点击事件
if (e.getSource() == addButton) {
// 添加学生信息
String name = nameField.getText();
int age = Integer.parseInt(ageField.getText());
String gender = maleRadioButton.isSelected() ? "男" : "女";
String department = (String) departmentComboBox.getSelectedItem();
// TODO: 将学生信息添加到数据库中
} else if (e.getSource() == searchButton) {
// 查询学生信息
String name = nameField.getText();
// TODO: 从数据库中查询学生信息,并显示在界面上
} else if (e.getSource() == modifyButton) {
// 修改学生信息
String name = nameField.getText();
int age = Integer.parseInt(ageField.getText());
String gender = maleRadioButton.isSelected() ? "男" : "女";
String department = (String) departmentComboBox.getSelectedItem();
// TODO: 将学生信息修改到数据库中
} else if (e.getSource() == deleteButton) {
// 删除学生信息
String name = nameField.getText();
// TODO: 从数据库中删除学生信息
}
}
public static void main(String[] args) {
new StudentInfoGUI();
}
}
```
UML类图文本:
```
+-----------------------+
| StudentInfoGUI |
+-----------------------+
| -nameLabel: JLabel |
| -genderLabel: JLabel |
| -ageLabel: JLabel |
| -departmentLabel: JLabel |
| -nameField: JTextField |
| -ageField: JTextField |
| -maleRadioButton: JRadioButton |
| -femaleRadioButton: JRadioButton |
| -departmentComboBox: JComboBox<String> |
| -addButton: JButton |
| -searchButton: JButton |
| -modifyButton: JButton |
| -deleteButton: JButton |
+-----------------------+
| +actionPerformed(ActionEvent e): void |
+-----------------------+
UML类图用Visio实现:
1. 打开Visio,选择“软件”分类,选择“UML类图”模板。
2. 点击左侧工具栏中的“类”图形,拖动到画布上并放置到适当的位置。
3. 双击类图形,在弹出的对话框中输入类名“StudentInfoGUI”并点击OK。
4. 点击左侧工具栏中的“属性”图形,拖动到类图形中并放置到适当的位置。
5. 双击属性图形,在弹出的对话框中输入属性名称并选择属性类型,并点击OK。
6. 重复步骤4-5,添加类的所有属性。
7. 点击左侧工具栏中的“操作”图形,拖动到类图形中并放置到适当的位置。
8. 双击操作图形,在弹出的对话框中输入操作名称并选择返回类型,并点击OK。
9. 重复步骤7-8,添加类的所有操作。
10. 连接类图形中的属性和操作,形成类的结构。
11. 保存UML类图文件。
相关推荐
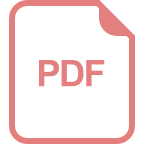
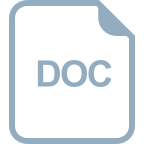
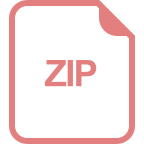














