死锁避免算法的实现 编写实现银行家算法,在算法执行过程中,首先判断申请资源的进程所申请的资源数目是否合法,若合法,则可以为其进行试分配,再利用安全性算法判断是否存在安全序列,若存在,则说明可以给申请资源的进程分配资源。否则,不进行资源的分配。用Java实现
时间: 2024-02-19 20:03:15 浏览: 45
以下是用Java实现银行家算法的代码:
```java
import java.util.Scanner;
public class BankerAlgorithm {
private int[][] allocation; // 分配矩阵
private int[][] max; // 最大需求矩阵
private int[][] need; // 需求矩阵
private int[] available; // 可用资源向量
private int processNum; // 进程数量
private int resourceNum; // 资源数量
private boolean[] finish; // 完成进程数组
public BankerAlgorithm(int[][] allocation, int[][] max, int[] available) {
this.allocation = allocation;
this.max = max;
this.available = available;
processNum = allocation.length;
resourceNum = available.length;
need = new int[processNum][resourceNum];
finish = new boolean[processNum];
// 初始化 need 矩阵和 finish 数组
for (int i = 0; i < processNum; i++) {
for (int j = 0; j < resourceNum; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
finish[i] = false;
}
}
// 判断是否存在安全序列
public boolean isSafe() {
int[] work = available.clone(); // 可用资源向量的副本
boolean[] tempFinish = finish.clone(); // 完成进程数组的副本
int count = 0; // 已完成进程数
int[] safeSequence = new int[processNum]; // 安全序列
while (count < processNum) {
boolean found = false;
for (int i = 0; i < processNum; i++) {
if (!tempFinish[i]) {
boolean enoughResource = true;
for (int j = 0; j < resourceNum; j++) {
if (need[i][j] > work[j]) {
enoughResource = false;
break;
}
}
if (enoughResource) {
for (int j = 0; j < resourceNum; j++) {
work[j] += allocation[i][j];
}
tempFinish[i] = true;
safeSequence[count++] = i;
found = true;
}
}
}
if (!found) {
return false; // 不存在安全序列
}
}
return true; // 存在安全序列
}
// 分配资源
public boolean requestResources(int processId, int[] request) {
for (int i = 0; i < resourceNum; i++) {
if (request[i] > need[processId][i] || request[i] > available[i]) {
return false; // 申请资源数目不合法
}
}
for (int i = 0; i < resourceNum; i++) {
available[i] -= request[i];
allocation[processId][i] += request[i];
need[processId][i] -= request[i];
}
if (isSafe()) {
return true; // 分配资源成功
} else {
// 回滚分配
for (int i = 0; i < resourceNum; i++) {
available[i] += request[i];
allocation[processId][i] -= request[i];
need[processId][i] += request[i];
}
return false; // 分配资源失败
}
}
public static void main(String[] args) {
int[][] allocation = {{2, 0, 1}, {3, 2, 0}, {1, 2, 2}, {0, 1, 2}, {0, 0, 2}};
int[][] max = {{3, 2, 2}, {6, 1, 3}, {3, 1, 4}, {6, 2, 1}, {4, 3, 1}};
int[] available = {3, 3, 2};
BankerAlgorithm bankerAlgorithm = new BankerAlgorithm(allocation, max, available);
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("请输入进程编号和申请资源数目:");
int processId = scanner.nextInt();
int[] request = new int[3];
for (int i = 0; i < 3; i++) {
request[i] = scanner.nextInt();
}
if (bankerAlgorithm.requestResources(processId, request)) {
System.out.println("分配资源成功!");
} else {
System.out.println("分配资源失败!");
}
}
}
}
```
上述代码中,`BankerAlgorithm` 类表示银行家算法。在类的构造函数中,传入分配矩阵、最大需求矩阵和可用资源向量,并根据它们计算出需求矩阵和完成进程数组的初始值。`isSafe` 方法用于判断是否存在安全序列,采用银行家算法的思想,从未完成的进程中找到一个可以完成的进程,并将其加入安全序列中,直到所有进程都已经完成或者没有可以完成的进程。`requestResources` 方法用于分配资源,首先判断申请资源数目是否合法,若合法则进行试分配,并判断是否存在安全序列。如果存在安全序列,则说明分配资源成功;否则,回滚分配,并说明分配资源失败。在 `main` 方法中,不断从标准输入中读取进程编号和申请资源数目,并调用 `requestResources` 方法进行资源的分配。
阅读全文
相关推荐
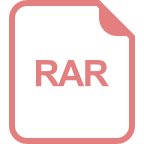
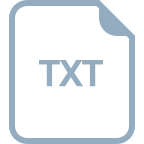

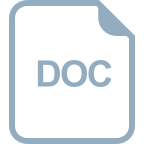
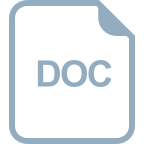
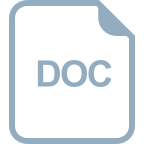
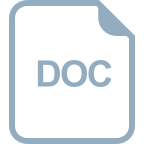
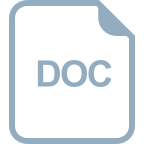
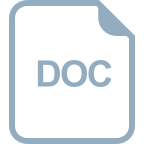
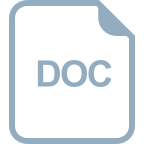
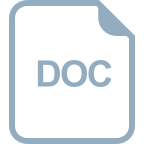
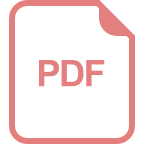
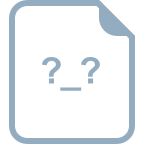
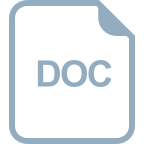


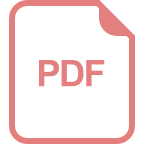