利用 Python 软件进行 LDA 主题分类,运算当主题数为多少时困惑度达到最低,确定文本的最优主题数,得到每篇文档的概率生成分布
时间: 2023-08-10 19:03:39 浏览: 70
首先,你需要安装并导入 gensim 库和 pyLDAvis 库。然后,你可以按照以下步骤进行 LDA 主题分类:
1. 加载语料库并创建字典和词袋模型:
```python
from gensim import corpora, models
# 加载语料库
corpus = corpora.TextCorpus('path/to/corpus')
# 创建字典
dictionary = corpus.dictionary
# 创建词袋模型
bow_corpus = [dictionary.doc2bow(doc) for doc in corpus]
```
2. 训练 LDA 模型并计算困惑度:
```python
from gensim.models import CoherenceModel, LdaModel
# 训练 LDA 模型
model_list = []
for num_topics in range(2, 11):
lda_model = LdaModel(bow_corpus, num_topics=num_topics, id2word=dictionary)
model_list.append(lda_model)
# 计算困惑度
coherence_scores = []
for model in model_list:
coherence_model = CoherenceModel(model=model, corpus=bow_corpus, coherence='u_mass')
coherence_score = coherence_model.get_coherence()
coherence_scores.append(coherence_score)
```
3. 找到困惑度最低的主题数:
```python
import matplotlib.pyplot as plt
# 绘制困惑度随主题数变化的曲线
num_topics = range(2, 11)
plt.plot(num_topics, coherence_scores)
plt.xlabel("Number of Topics")
plt.ylabel("Coherence score")
plt.show()
# 找到困惑度最低的主题数
best_num_topics = num_topics[coherence_scores.index(min(coherence_scores))]
print("Best number of topics:", best_num_topics)
```
4. 使用最优主题数重新训练 LDA 模型并得到每篇文档的概率生成分布:
```python
# 训练最优主题数的 LDA 模型
best_lda_model = LdaModel(bow_corpus, num_topics=best_num_topics, id2word=dictionary)
# 得到每篇文档的概率生成分布
doc_topic_distribution = []
for doc in bow_corpus:
doc_topic_distribution.append(best_lda_model.get_document_topics(doc))
```
相关推荐
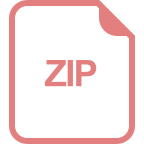
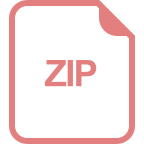
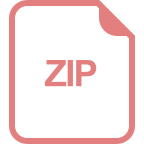














