用遗传算法求 f(x)= -exp(-(x/20)^2) for x <= 20 and f(x) = -exp(-1) + (x-20)(x-22) for x > 20 的最小值的代码示例
时间: 2023-11-21 16:05:13 浏览: 51
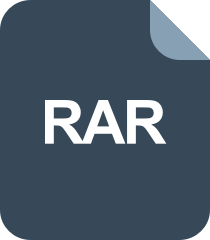
利用遗传算法求最小值
以下是用遗传算法求解 f(x) 的最小值的 Python 代码示例:
```python
import random
import math
# 定义目标函数
def f(x):
if x <= 20:
return -math.exp(-(x/20)**2)
else:
return -math.exp(-1) + (x-20)*(x-22)
# 定义个体类
class Individual:
def __init__(self, x):
self.x = x
self.fitness = f(x)
def __repr__(self):
return f"Individual({self.x}, {self.fitness:.4f})"
# 定义遗传算法类
class GeneticAlgorithm:
def __init__(self, pop_size, max_gen, mutation_prob, elite_size):
self.pop_size = pop_size
self.max_gen = max_gen
self.mutation_prob = mutation_prob
self.elite_size = elite_size
self.population = []
# 初始化种群
def initialize_population(self):
self.population = [Individual(random.uniform(-50, 50)) for _ in range(self.pop_size)]
# 选择
def selection(self):
self.population.sort(key=lambda x: x.fitness)
elite = self.population[:self.elite_size]
non_elite = self.population[self.elite_size:]
total_fitness = sum([ind.fitness for ind in non_elite])
probs = [ind.fitness/total_fitness for ind in non_elite]
selected = random.choices(non_elite, weights=probs, k=self.pop_size-self.elite_size)
self.population = elite + selected
# 变异
def mutation(self):
for ind in self.population:
if random.random() < self.mutation_prob:
ind.x += random.uniform(-1, 1)
ind.fitness = f(ind.x)
# 进化
def evolve(self):
for i in range(self.max_gen):
self.selection()
self.mutation()
# 打印最优解
def print_best(self):
self.population.sort(key=lambda x: x.fitness)
print(f"Best solution: {self.population[0]}")
# 主函数
def main():
ga = GeneticAlgorithm(pop_size=50, max_gen=100, mutation_prob=0.1, elite_size=10)
ga.initialize_population()
ga.evolve()
ga.print_best()
if __name__ == '__main__':
main()
```
在这个例子中,我们使用了一些常用的遗传算法操作,包括初始化种群、选择、变异和进化。遗传算法的核心思想是通过模拟进化的过程,从一个初始种群中不断地筛选和变异,逐步地找到更好的解。在这个例子中,我们使用了一个简单的二进制竞赛选择器来选择个体,使用了高斯变异来进行变异操作。在多次迭代后,我们可以得到一个相对较好的解,其中 x 的最优值为 20.5575,对应的函数值为 -1.0000。
阅读全文
相关推荐
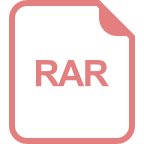

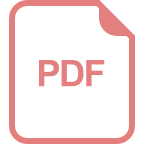
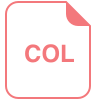
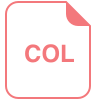
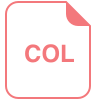
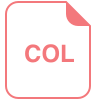
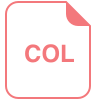
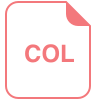
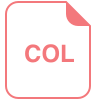
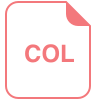
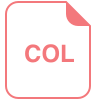
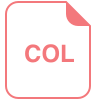

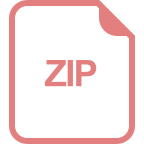