上面代码需要添加头文件吧
时间: 2024-02-21 09:56:57 浏览: 73
是的,这段代码需要添加一些头文件,如下所示:
```c
#include <stdio.h>
#include <curl/curl.h>
```
其中,`stdio.h`是标准输入输出头文件,用于输出错误信息;`curl/curl.h`是libcurl库的头文件,包含了libcurl库的所有函数声明和定义。如果编译器找不到这些头文件,需要在编译选项中加入相关的库文件路径和库文件名。
相关问题
你上面这段代码需要包含头文件吗
是的,上述代码需要包含头文件`<iomanip>`,该头文件中定义了`setw`和`setiosflags`函数。因此,在使用这两个函数之前,需要在代码中添加以下头文件引用:
```c++
#include <iomanip>
```
这样就可以正确使用`setw`和`setiosflags`函数了。
在上述代码之前定义一个会使用到的SequenceList模板类,还有所有代码需要的头文件
在开始具体的函数实现前,我们首先定义一个名为`SequenceList`的模板类,它存储任意类型的元素,并提供了一些基本的操作。这里包含一个简单的容器以及一些必要的成员函数。为了简化代码,我们假设`SequenceList`支持迭代器操作和自增。
```cpp
// sequence_list.h
#ifndef SEQUENCE_LIST_H_
#define SEQUENCE_LIST_H_
#include <iostream>
#include <vector>
#include <stdexcept>
#include <algorithm>
template <typename T>
class SequenceList {
public:
SequenceList() : items() {}
explicit SequenceList(size_t initial_size) : items(initial_size, T{}) {}
~SequenceList() {}
// 添加元素
void push_back(const T& value) {
items.push_back(value);
}
// 插入元素
void insert_at(size_t pos, const T& value) {
items.insert(items.begin() + pos, value);
}
// 获取元素
const T& operator[](size_t index) const {
if (index >= size()) {
throw std::out_of_range("Index out of range.");
}
return items[index];
}
// 遍历
iterator begin() { return items.begin(); }
iterator end() { return items.end(); }
// 获得元素数量
size_t size() const { return items.size(); }
private:
std::vector<T> items;
};
// 简单的自定义迭代器
template <typename T>
class SequenceListIterator {
friend class SequenceList<T>;
using value_type = T;
public:
SequenceListIterator(const SequenceList<T>& list, size_t index)
: list_(list), current_index_(index) {}
value_type operator*() const {
return list_[current_index_];
}
SequenceListIterator& operator++() {
++current_index_;
return *this;
}
bool operator==(const SequenceListIterator& other) const {
return current_index_ == other.current_index_;
}
bool operator!=(const SequenceListIterator& other) const {
return !(*this == other);
}
private:
const SequenceList<T>& list_;
size_t current_index_;
};
using iterator = SequenceListIterator<int>;
#endif // SEQUENCE_LIST_H_
```
上面的代码包含了`SequenceList`的基本构造、添加元素、获取元素、遍历以及一些辅助迭代器。接下来你可以使用这个类来实现前面提到的函数。记得包含`sequence_list.h`文件。在这个基础上,你可以继续询问关于这个类的使用或者其他相关问题。
阅读全文
相关推荐
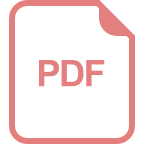
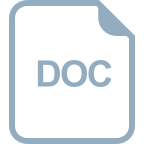











