linux tcpdump C++ code
时间: 2023-10-05 17:13:00 浏览: 64
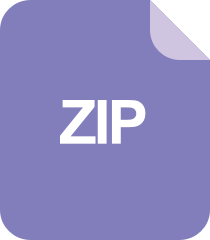
C++生成dump文件
Here is an example of a simple C program to use tcpdump on Linux:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <errno.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <netinet/ip.h>
#include <netinet/tcp.h>
#define BUFSIZE 1024
int main(int argc, char *argv[]) {
int sockfd, n;
char buffer[BUFSIZE];
struct sockaddr_in servaddr;
struct iphdr *ip;
struct tcphdr *tcp;
if (argc != 2) {
printf("Usage: %s <interface>\n", argv[0]);
exit(1);
}
if ((sockfd = socket(AF_INET, SOCK_RAW, IPPROTO_TCP)) < 0) {
perror("socket");
exit(1);
}
memset(&servaddr, 0, sizeof(servaddr));
servaddr.sin_family = AF_INET;
servaddr.sin_addr.s_addr = htonl(INADDR_ANY);
servaddr.sin_port = htons(0);
if (bind(sockfd, (struct sockaddr *)&servaddr, sizeof(servaddr)) < 0) {
perror("bind");
exit(1);
}
printf("Listening on interface %s...\n", argv[1]);
while (1) {
n = recvfrom(sockfd, buffer, BUFSIZE, 0, NULL, NULL);
if (n < 0) {
perror("recvfrom");
exit(1);
}
ip = (struct iphdr *) buffer;
tcp = (struct tcphdr *) (buffer + sizeof(struct iphdr));
printf("Source IP: %s\n", inet_ntoa(*(struct in_addr *)&ip->saddr));
printf("Source port: %d\n", ntohs(tcp->source));
printf("Destination IP: %s\n", inet_ntoa(*(struct in_addr *)&ip->daddr));
printf("Destination port: %d\n", ntohs(tcp->dest));
printf("\n");
}
close(sockfd);
return 0;
}
```
This program creates a raw socket and binds it to the specified interface. It then listens for incoming TCP packets and prints out the source and destination IP addresses and port numbers. Note that this program requires root privileges to run.
阅读全文
相关推荐
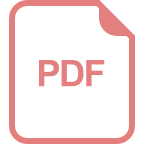
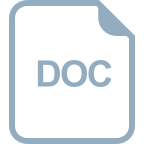
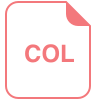
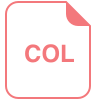
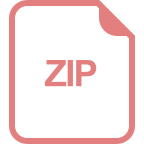
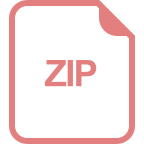
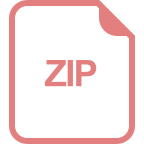
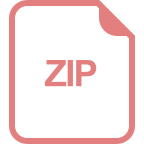
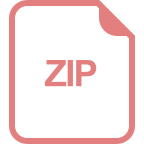
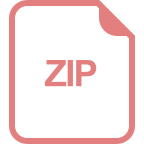
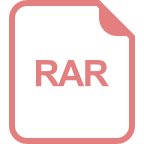