Java来实现编写一个图形界面程序实现自动投掷筛子100次的统计,在窗体中显示投掷过程,显 示投掷的值,每隔1秒投掷1次,点击投掷按钮开始投掷。 利用多线程实现自动投掷。最后,通过对话框显示投掷结果。 可增加一个按钮控制投掷过程的中止。中止时显示目前投掷的次数,各个值统计占比 情况
时间: 2023-12-16 09:03:23 浏览: 33
以下是Java实现自动投掷筛子100次的统计的代码:
```java
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class DiceRoller extends JFrame {
private static final long serialVersionUID = 1L;
private JButton btnRoll;
private JButton btnStop;
private JPanel panel;
private JTextField tfResult;
private JLabel[] lblValues;
private JLabel[] lblPercentage;
private int[] values;
private int totalRolls;
private boolean rolling;
private Thread thread;
public DiceRoller() {
setTitle("Dice Roller");
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
panel = new JPanel();
btnRoll = new JButton("Roll Dice");
btnRoll.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
rollDice();
}
});
btnStop = new JButton("Stop Rolling");
btnStop.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
stopRolling();
}
});
tfResult = new JTextField(20);
panel.add(btnRoll);
panel.add(btnStop);
panel.add(tfResult);
add(panel, BorderLayout.NORTH);
JPanel panelValues = new JPanel();
panelValues.setLayout(new BorderLayout());
panelValues.add(new JLabel("Value"), BorderLayout.WEST);
panelValues.add(new JLabel("Percentage"), BorderLayout.EAST);
panelValues.add(new JLabel(" "), BorderLayout.CENTER);
lblValues = new JLabel[6];
lblPercentage = new JLabel[6];
values = new int[6];
for (int i = 0; i < 6; i++) {
values[i] = 0;
lblValues[i] = new JLabel(String.valueOf(i + 1));
lblPercentage[i] = new JLabel("0%");
JPanel panelValue = new JPanel();
panelValue.setLayout(new BorderLayout());
panelValue.add(lblValues[i], BorderLayout.WEST);
panelValue.add(lblPercentage[i], BorderLayout.EAST);
panelValues.add(panelValue, BorderLayout.SOUTH);
}
add(panelValues, BorderLayout.CENTER);
setVisible(true);
rolling = false;
}
private void rollDice() {
rolling = true;
totalRolls = 0;
for (int i = 0; i < 6; i++) {
values[i] = 0;
}
thread = new Thread() {
public void run() {
Random rand = new Random();
while (rolling) {
int value = rand.nextInt(6) + 1;
values[value - 1]++;
totalRolls++;
tfResult.setText(String.valueOf(value));
updatePercentage();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
};
thread.start();
}
private void stopRolling() {
rolling = false;
thread.interrupt();
String message = "Total Rolls: " + totalRolls + "\n";
for (int i = 0; i < 6; i++) {
double percentage = ((double) values[i] / totalRolls) * 100;
message += "Value: " + (i + 1) + ", Count: " + values[i] + ", Percentage: " + String.format("%.2f", percentage) + "%\n";
}
JOptionPane.showMessageDialog(this, message);
}
private void updatePercentage() {
for (int i = 0; i < 6; i++) {
double percentage = ((double) values[i] / totalRolls) * 100;
lblPercentage[i].setText(String.format("%.2f", percentage) + "%");
}
}
public static void main(String[] args) {
new DiceRoller();
}
}
```
在该程序中,使用了多线程技术实现自动投掷筛子。点击“Roll Dice”按钮后,程序会启动一个新线程,在该线程中不断生成随机数模拟投掷筛子,并更新界面显示和统计结果。点击“Stop Rolling”按钮后,程序会中止投掷过程,并弹出一个对话框显示投掷结果。
相关推荐
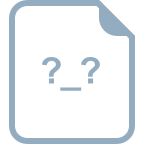














