vector<vector<string>> a,如何删去字符串中的某个字符
时间: 2023-09-24 12:11:04 浏览: 95
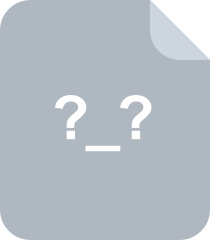
删除字符串中某一字符
如果你想要删除字符串中的某个字符,可以使用字符串的 erase() 方法。
假设你想要删除 a[i][j] 中的第 k 个字符,你可以使用下面的代码:
```cpp
a[i][j].erase(k, 1);
```
其中,`k` 表示要删除的字符的下标,`1` 表示要删除的字符数(因为你只删除一个字符,所以这里是 1)。
需要注意的是,这个方法会改变原字符串,所以如果你想要保留原字符串,可以先将其复制一份,然后在复制的字符串上进行操作。
阅读全文
相关推荐















