def Focal_Loss(inputs, target, cls_weights, num_classes=21, alpha=0.5, gamma=2): n, c, h, w = inputs.size() nt, ht, wt = target.size() if h != ht and w != wt: inputs = F.interpolate(inputs, size=(ht, wt), mode="bilinear", align_corners=True) temp_inputs = inputs.transpose(1, 2).transpose(2, 3).contiguous().view(-1, c) temp_target = target.view(-1) logpt = -nn.CrossEntropyLoss(weight=cls_weights, ignore_index=num_classes, reduction='none')(temp_inputs, temp_target) pt = torch.exp(logpt) if alpha is not None: logpt *= alpha loss = -((1 - pt) ** gamma) * logpt loss = loss.mean() return loss 是什么意思
时间: 2023-10-07 18:13:30 浏览: 293
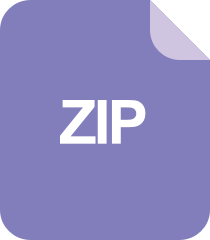
focal_loss_visualization
这段代码实现了 Focal Loss,是一种用于解决分类问题中,样本不平衡导致的分类精度下降的方法。其中,输入的参数包括:
- inputs:模型的输出,是一个大小为 (n, c, h, w) 的张量,其中 n 表示 batch size,c 表示类别数,h 和 w 表示输入图片的高和宽。
- target:真实标签,是一个大小为 (n, h, w) 的张量。
- cls_weights:各个类别的权重,是一个大小为 (c,) 的张量。
- num_classes:类别数,默认为 21。
- alpha:平衡因子,控制正负样本的权重比例,默认为 0.5。
- gamma:调节因子,控制易错样本的权重,默认为 2。
该函数的返回值是一个标量,表示 Focal Loss 的值。
阅读全文
相关推荐
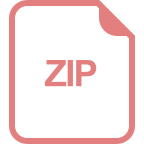
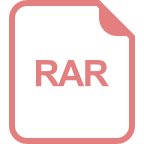


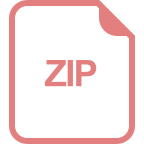
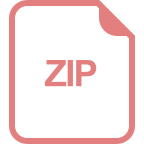
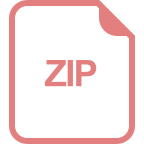
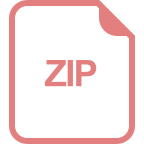
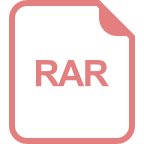
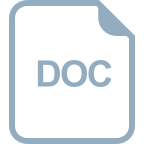








