c语言实现二叉树链查找数据,其中的二叉树链没有排序规律
时间: 2023-11-14 12:10:34 浏览: 71
题目:c语言实现二叉树链查找数据,其中的二叉树链没有排序规律。
可以使用以下代码实现:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
int data;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
TreeNode* createNode(int value) {
TreeNode* node = malloc(sizeof(TreeNode));
node->data = value;
node->left = NULL;
node->right = NULL;
return node;
}
void insert(TreeNode** root, int value) {
if (*root == NULL) {
*root = createNode(value);
return;
}
if (value < (*root)->data) {
insert(&(*root)->left, value);
} else {
insert(&(*root)->right, value);
}
}
int search(TreeNode* root, int value) {
if (root == NULL) {
return 0;
}
if (value == root->data) {
return 1;
}
if (value < root->data) {
return search(root->left, value);
} else {
return search(root->right, value);
}
}
int main() {
TreeNode* root = NULL;
insert(&root, 5);
insert(&root, 8);
insert(&root, 2);
insert(&root, 6);
insert(&root, 1);
insert(&root, 9);
int isFound = search(root, 6);
if (isFound) {
printf("The value is found in the binary tree.\n");
} else {
printf("The value is not found in the binary tree.\n");
}
return 0;
}
```
此代码使用二叉树链实现查找数据,函数中没有规定排序规律,因此可以处理非有序的查找数据。
阅读全文
相关推荐
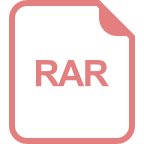





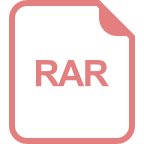








